Published on May 24, 2025
Dart Tutorial: Complete Guide for Beginners
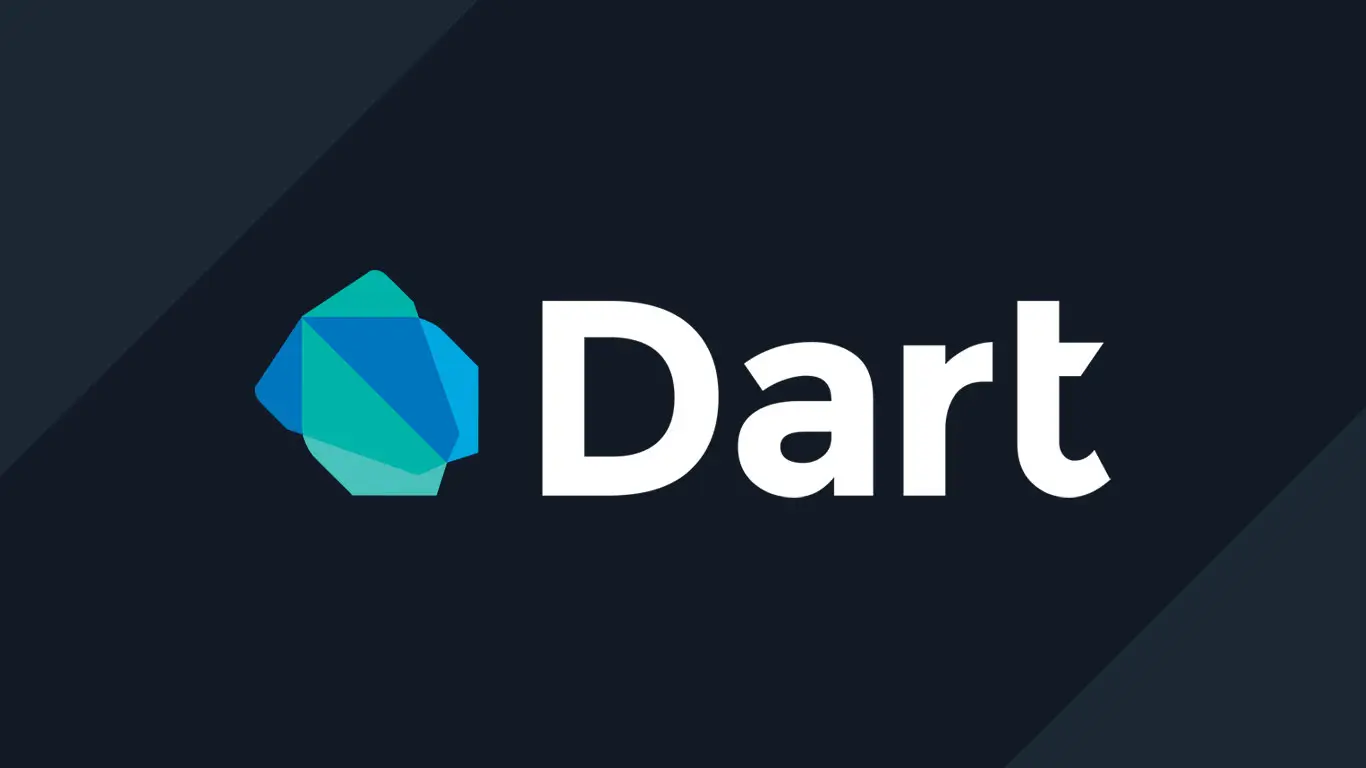
Dart is a modern programming language developed by Google and widely used to build cross-platform applications with Flutter. If you want to create fast and efficient mobile, web, or even backend applications, then Dart is the perfect choice.
In this article, we will discuss a complete Dart language tutorial, from basic introduction to using important features. Suitable for those of you who are just starting to code or who want to expand your skills to the realm of Flutter and beyond.
What is the Dart Programming Language?
Dart is a statically typed, object-oriented programming language with syntax similar to JavaScript and Java . This language was originally designed to replace JavaScript in web development, but is now growing rapidly thanks to its integration with Flutter.
Advantages of Dart:
- Compatible with Flutter
- High performance (compiled to native code)
- Easy to learn
- Clean syntax
- Async/await support for asynchronous programming
Installing Dart on a Computer
Before you start writing Dart code, you need to install it first. You can use the Dart SDK separately or directly through the Flutter SDK (if you want to develop a Flutter application).
- Dart SDK Installation:
- Visit the official website: https://dartpad.dev
- Select your operating system (Windows, macOS, or Linux)
- Follow the installation instructions
- Check the installation with the command:
dart --version
Basic Dart Program Structure
Here is an example of the simplest Dart program:
void main() {
print('Hello, Dart!');
}
Explanation:
void main()
is the entry point of the program.print()
is a function to print text to the console.
Variables and Data Types in Dart
Dart is a language with static data types, but you can also use the var
keyword for dynamic data types.
int angka = 10;
double pi = 3.14;
String nama = 'Syukra';
bool aktif = true;
var dynamicData = 'Can change type';
Program Flow Control
Branching:
if (angka > 5) {
print('Number is greater than 5');
} else {
print('Number is 5 or less');
}
Looping:
for (int i = 0; i < 5; i++) {
print('Iteration ke-$i');
}
Functions in Dart
Functions are essential in Dart to make code more modular and structured.
int add(int a, int b) {
return a + b;
}
void main() {
print(add(5, 3)); // Output: 8
}
Null Safety
Dart supports null safety, which means you have to explicitly specify whether a variable can be null or not.
String? nama; // Can be null
String namaLain = 'Not null'; // Cannot be null
This feature helps prevent bugs and errors at runtime.
Lists, Sets, and Maps
- Lists (like arrays):
List<String> buah = ['Apple', 'Orange', 'Mango'];
print(buahan[0]); // Apel
- Set (unique elements):
Set<int> angka = {1, 2, 3, 3};
print(angka); // {1, 2, 3}
- Map (key-value):
Map<String, String> biodata = {
'nama': 'Syukra',
'kota': 'Bandung'
};
print(biodata['name']); // Syukra
Object-Oriented Programming (OOP)
Dart supports OOP principles such as class, inheritance, encapsulation, and polymorphism.
class Vehicle {
String merk;
Vehicle(this.merk);
void info() {
print('Merk: $merk');
}
}
void main() {
var mobil = Kendaraan('Toyota');
mobil.info();
}
Asynchronous Programming
Dart is perfect for modern applications because it supports asynchronous operations.
Future<void> fetchData() async {
await Future.delayed(Duration(seconds: 2));
print('Data successfully fetched');
}
void main() {
fetchData();
print('Waiting for data...');
}
Using Dart in Flutter
Dart is the primary language for building UI in Flutter. Here’s an example of a simple widget:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Hello Flutter')),
body: Center(child: Text('Learn Dart for Flutter')),
),
);
}
}
Recommended Tools & Editors
- VS Code: lightweight and flexible
- Android Studio: complete for Flutter
- DartPad: official online editor at https://dartpad.dev
Tips for Learning Dart Quickly
- Start with the official documentation at https://dartpad.dev
- Practice directly on DartPad
- Learn Flutter for real applications
- Use the
debug
andbreakpoint
features - Join the Dart & Flutter community on Telegram or Discord
Conclusion
Dart is a flexible, fast programming language, and is directly supported by Google . Suitable for anyone who wants to build multiplatform applications with Flutter. By learning the basics of Dart as in this tutorial, you are one step closer to becoming a modern developer who is ready to face the challenges of the digital world.