Published on Dec 18, 2024
Last updated on Apr 11, 2025
Easy Guide to Handling Bugs in Software Development
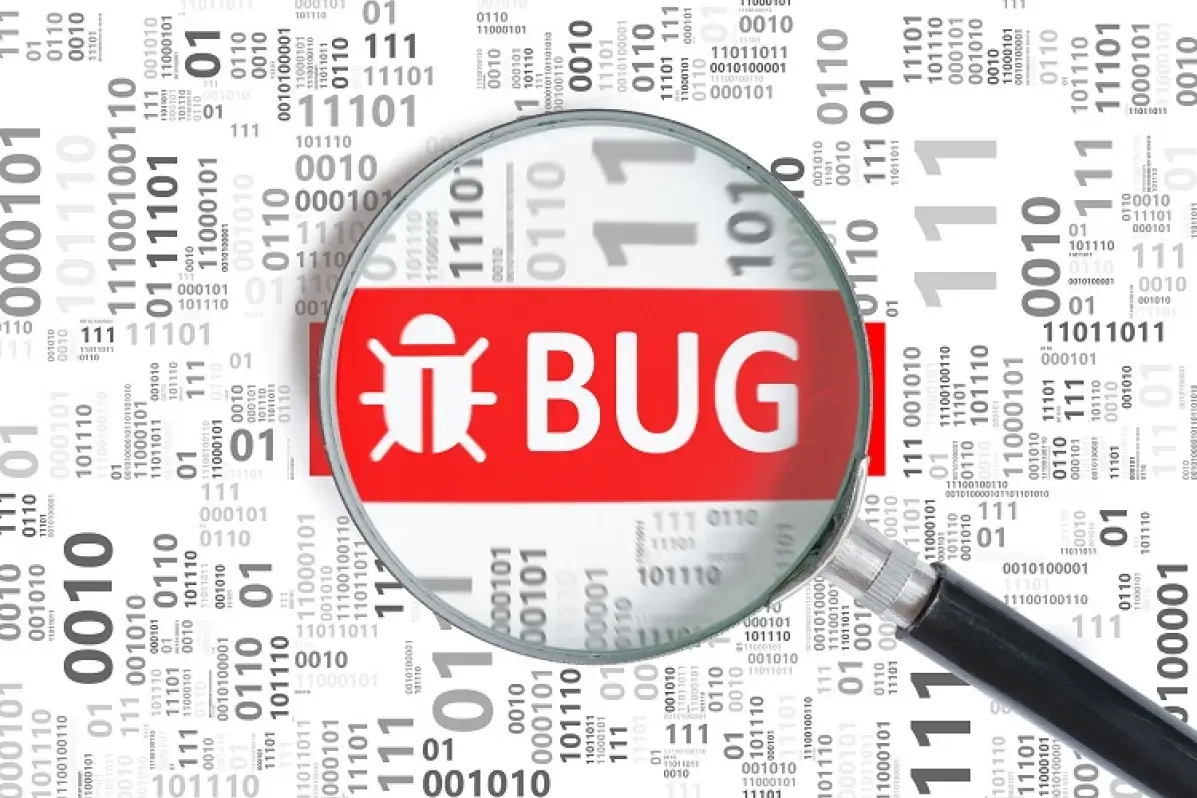
In software development, bugs are a common problem that is often encountered. Bugs can range from minor issues that do not have a significant impact to major problems that can stop the functionality of the system. Effectively handling bugs is key to maintaining software quality and user satisfaction. This article will discuss effective ways to handle bugs, from identification to fixing and testing.
1. Bug Identification
- Users as a Source of Information
Often, bugs are first reported by users. It is important to listen to their feedback and analyze the reports they provide. Document the steps that users took before the bug occurred, as well as the hardware and software environment conditions used.
- Automated and Manual Testing
Automated testing using tools such as unit tests or integration tests can help find bugs before the software is released. However, manual testing is also needed to identify problems that may not be detected by automated tests.
2. Bug Reproduction
Once a bug has been identified, the next step is to try to reproduce the problem. This helps in understanding the conditions under which the bug occurs and narrows down the search area for a solution. Document the reproduction steps clearly to facilitate the debugging process.
3. Analysis and Diagnostics
- Code Analysis
Examine the relevant code to understand why the bug occurred. Use monitoring and debugging tools to trace the execution flow and find the point where the error occurred.
- Logs and Error Messages
Review the application logs and error messages for additional information that can help diagnose the problem. This often provides clues as to what went wrong and where.
4. Bug Fixing
- Code Modification
Once you find the cause of the bug, make changes to the code to fix it. Make sure the changes are thoroughly tested to ensure no new issues are introduced.
- Code Review
Perform a code review after the fix to ensure the quality and integrity of the code is maintained. Involving other teams in the review can help catch potential issues that might have been missed.
5. Retesting and Verification
- Testing After Fixing
Retest to ensure that the bug has been fixed and that no new issues have been introduced as a result of the fix. Use the same test scenario that was used to recreate the bug.
- Full Coverage Testing
In addition to retesting, perform full coverage testing to ensure that the fix does not impact other parts of the application.
6. Documentation and Reporting
- Fix Documentation
Record all details about the bug, including how it was discovered, the steps to fix it, and the test results. This documentation is important for future reference and to help other teams who may encounter similar issues.
- Reporting to Users
If the fixed bug impacts end users, let them know about the fix through release notes or a software update. Transparency in communication can increase user trust.
What are Examples of Common Bugs?
Here are some examples of common bugs that often occur in software development:
1. Null Pointer Exception
This bug occurs when an application tries to access or manipulate an object that has not been initialized (null). For example, if a method tries to call a method on an object that has not been set, a Null Pointer Exception will occur.
Case Example:
String message = null;
int length = message.length(); // Null Pointer Exception
Solution: Always make sure the object is initialized before use and add null checks in relevant places.
2. Array Index Out of Bounds
This bug occurs when an application tries to access an array element with an index that is outside the valid range. This often occurs when the index calculation is inaccurate.
Case Example:
int[] numbers = {1, 2, 3};
int value = numbers[5]; // Array Index Out of Bounds Exception
Solution: Verify that the index is within a valid range before accessing the array element.
3. Memory Leak
A memory leak occurs when an application does not release memory that is no longer in use. This can cause the application to use more memory than necessary and eventually crash or slow down.
Case Study:
List<String> list = new ArrayList<>();
while (true) {
list.add(new String("Memory Leak")); // Memory Leak
}
Solution: Be sure to release unused resources and consider using a memory monitoring tool to detect memory leaks.
4. Race Condition
A race condition occurs when two or more processes or threads access a shared resource without proper synchronization, which can lead to undesired behavior or inconsistent results.
Case Study:
public class Counter {
private int count = 0;
public void increment() {
count++;
}
}
If the increment()
method is called by multiple threads concurrently, the results can be inconsistent.
Solution: Use a synchronization mechanism such as synchronized
block or java.util.concurrent
classes to ensure safe access to shared resources.
5. Infinite Loop
Infinite loop occurs when code enters a loop that never terminates. This is often caused by the loop condition never being false.
Case Example:
while (true) {
System.out.println("This will run forever");
}
Solution: Make sure there is a proper condition to exit the loop and check the loop logic to make sure the condition stops.
6. Off-by-One Error
This bug occurs when an error occurs in the calculation of loop boundaries or array indices, usually by accidentally leaving out or adding one element.
Case Example:
for (int i = 0; i <= 10; i++) {
System.out.println(i); // If the array has 10 elements, it will try to access the 11th element
}
Solution: Check the loop bounds and array indices carefully to ensure that no off-by-one errors occur.
7. Incorrect Calculation
This bug occurs when there is an error in the calculation logic or algorithm, resulting in inaccurate results.
Case Example:
int result = 10 / 3; // Might produce 3, when it should be 3.33
Solution: Verify the calculation logic and test to ensure that the results are as expected.
8. User Interface Glitches
These bugs involve problems with the user interface, such as elements not appearing correctly, broken layouts, or interactions not working.
Case Study:
<button>Click Me</button>
If CSS is not applied correctly, the button may not appear or function as expected.
Workaround: Test the user interface across multiple devices and screen resolutions to ensure consistent appearance and functionality.
9. Data Synchronization Issues
These bugs occur when data is not properly synchronized between different parts of an application or between an application and an external data source.
Case Study:
public class UserProfile {
private String name;
public void updateName(String newName) {
this.name = newName;
}
}
If name updates are not synchronized with the interface, users may see outdated data.
Solution: Implement proper data synchronization mechanisms and ensure updates are applied across the system.
10. Security Vulnerabilities
This bug relates to a security issue that could be exploited by an unauthorized party, such as SQL injection, XSS (Cross-Site Scripting), or sensitive data disclosure.
Case Example:
SELECT FROM users WHERE username = 'admin' OR '1'='1'; -- SQL Injection
Solution: Implement security best practices, such as input validation and use of parameterized queries to prevent exploits.
That’s all the articles from Admin, hopefully useful… Thank you for stopping by…