Fetch vs Axios: What is The Difference?
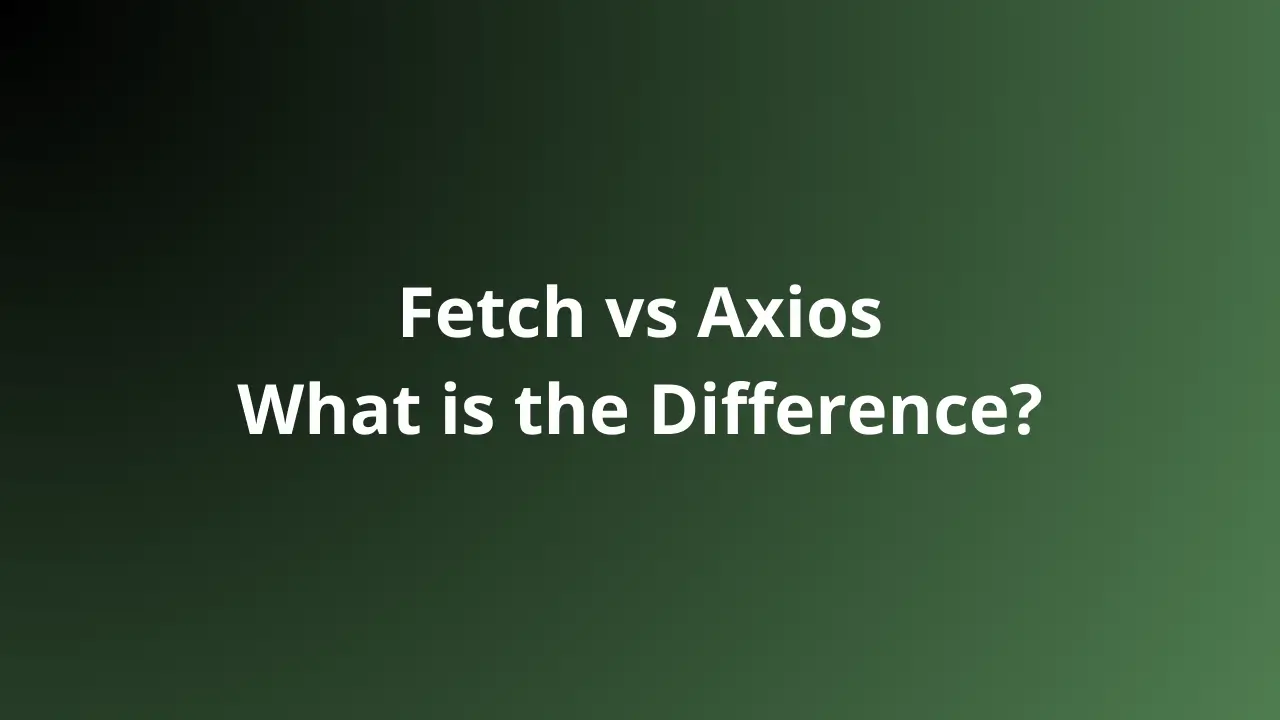
In modern web development, making HTTP requests is a common task, especially when interacting with APIs to fetch or send data. Two of the most commonly used tools for this purpose are Fetch API and Axios. While both serve the purpose of making HTTP requests, there are significant differences in terms of features, syntax, error handling, and browser compatibility. Understanding these differences is important for developers to choose the tool that best suits their project needs.
Introduction
In the JavaScript ecosystem, making HTTP requests is essential, especially when interacting with web services and APIs. Two commonly used tools for making HTTP requests are Fetch API and Axios. While both serve the same purpose, they offer different approaches and features. This article will discuss the differences between Fetch and Axios in detail, helping you understand when and why to choose one over the other.
What is Fetch API?
The Fetch API is a built-in JavaScript interface introduced in ECMAScript 6 (ES6) and is available in most modern browsers. This API provides a fetch()
method that allows you to perform network requests in a simpler and more structured way than previous approaches such as XMLHttpRequest
.
Example of Fetch API Usage:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network problem');
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('There was a problem with the fetch request:', error);
});
In the above example, fetch()
returns a Promise that will be resolved when the response is available. You need to check the ok
property of the response to ensure there are no HTTP errors, and then call the json()
method to parse the JSON data from the response.
What is Axios?
Axios is a Promise-based HTTP library that lets you make HTTP requests from a Node.js environment or from a browser. Axios offers advanced features such as request and response interception, request cancellation, response data transformation, and better error handling.
Axios Usage Example:
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('There was a problem with the axios request:', error);
});
In the above example, axios.get()
is used to make a GET request to the specified URL. The received response is already parsed into a JavaScript object, so you can directly access the data via the data
property.
Detailed Comparison between Fetch and Axios
Basic Syntax
The Fetch API uses a global function fetch()
that returns a Promise. To fetch JSON data, you need to call the json()
method on the response object.
Example with Fetch:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Axios provides methods like axios.get()
and axios.post()
that also return a Promise. The JSON data in the response is already parsed automatically.
Example with Axios:
axios.get('https://api.example.com/data')
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
The main difference here is that with Fetch, you need to call response.json()
to parse the data, whereas with Axios, the data is already available in response.data
.
Error Handling
In the Fetch API, requests are only rejected if there is a network error. HTTP errors, such as a 404 or 500 status, will not cause a Promise to be rejected. Therefore, you need to check the ok
property on the response to detect HTTP errors.
Example of Error Handling with Fetch:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
In contrast, Axios automatically rejects Promises for responses with status codes outside the 2xx range, making error handling much simpler.
Error Handling Example with Axios:
axios.get('https://api.example.com/data')
.then(response => console.log(response.data))
.catch(error => {
if (error.response) {
// Server responds with status outside 2