Published on May 24, 2025
How to Make a Weather Forecast App With Cpp
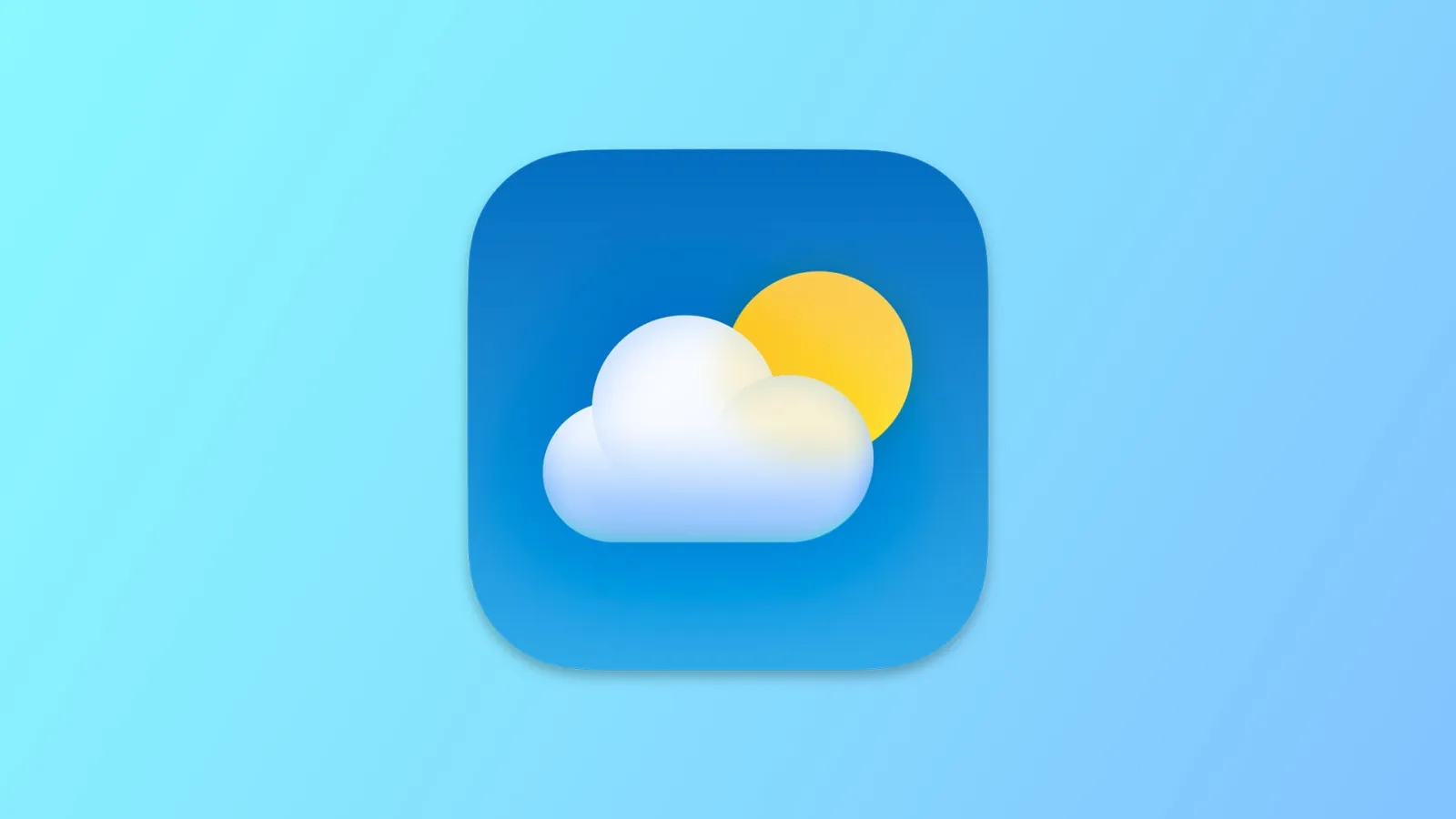
Weather forecast application is one of the simple yet very useful applications that can be developed using C++. With this application, we can display real-time weather information by accessing the API from the weather service. This article will explain how to create a simple weather forecast application using C++ completely, from installation to API data retrieval and displaying results. Let’s get started!
Preparation
Before we start writing code, make sure your computer has C++ installed and an IDE or text editor that supports C++ programming such as Code::Blocks, Visual Studio Code, or others.
a. Installing C++
If you don’t have C++ installed, you can download GCC Compiler from MinGW or Microsoft Visual Studio Community which provides a built-in C++ compiler.
b. Setting Up the Editor
Once C++ is ready, you can also choose a comfortable editor such as Visual Studio Code, then add the C/C++ extension to help debugging and see errors in the code writing.
Setting Up the Weather API
In order for the application to display current weather data, we need to access the weather API. Some popular weather API providers are OpenWeatherMap and Weatherstack. In this article, we will use OpenWeatherMap which provides free data for development.
a. Getting an API Key
- Register at OpenWeatherMap .
- After registering, go to the API page and select Current Weather Data.
- Note the API key provided because we will use it in the code.
b. API URL Format
The OpenWeatherMap API URL usually has the following format:
http://api.openweathermap.org/data/2.5/weather?q={city_name}&appid={API_key}&units=metric
Replace {city_name}
with the desired city name and {API_key}
with your API key.
Creating a Weather App in C++
Once the environment is ready and the API key is available, we will start by writing the code for the weather app.
Step 1: Initialize the Project
Create a new project file, for example WeatherApp.cpp
, and add the necessary libraries.
#include <iostream>
#include <string>
#include <curl/curl.h> // library to access the API via HTTP
using namespace std;
Here, we are using the curl
library to handle HTTP requests. Make sure you have libcurl
installed on your system.
Step 2: Function to Store API Data
We need a callback function to store the data received from the API. This function will store the data from the API into a string.
size_t WriteCallback(void contents, size_t size, size_t nmemb, void userp) {
((std::string)userp)->append((char)contents, size nmemb);
return size nmemb;
}
This WriteCallback
function will be called by curl
to store the API response in a string.
- Getting to Know RAM: Definition, Function, Types, and Important Roles in Computers
- Sinkclose Vulnerability: Definition, Causes, Impacts, and How to Overcome It
- The Complete Guide to Firebase Database
- Complete Guide to Using Cryptsetup on Kali Linux
- Is Antivirus Still Necessary in 2025? Experts Reveal the Truth
Step 3: Create the getWeatherData Function
Next, create the getWeatherData
function to fetch data from the API. This function will accept the city
and apiKey
parameters to access weather data from a particular city.
string getWeatherData(const string& city, const string& apiKey) {
CURL curl;
CURLcode res;
string readBuffer;
curl = curl_easy_init();
if (curl) {
string url = "http://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=" + apiKey + "&units=metric";
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
if (res != CURLE_OK)
cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << endl;
curl_easy_cleanup(curl);
}
return readBuffer;
}
This function:
- Initializes
curl
. - Constructs the API URL based on the city and API key.
- Sends an HTTP request and stores the result in
readBuffer
. - Returns the weather data in JSON format as a string.
Step 4: Parse JSON Data
Since the output of the API is JSON , we need a library to parse JSON, such as nlohmann/json. Download the JSON for C++ library, and add it to your project.
Add the following code for parsing:
#include "json.hpp"
using json = nlohmann::json;
Next, create a function parseWeatherData
to display the important weather data.
void parseWeatherData(const string& weatherData) {
auto jsonData = json::parse(weatherData);
string city = jsonData["name"];
double temperature = jsonData["main"]["temp"];
string weather = jsonData["weather"][0]["description"];
cout << "Kota: " << city << endl;
cout << "Suhu: " << temperature << "°C" << endl;
cout << "Cuaca: " << weather << endl;
}
This function:
- Parses JSON data using
json::parse
. - Displays important information such as city name, temperature, and weather description.
Step 5: Integrate Functions in main()
Next, create a main
function to run the application.
int main() {
string city;
string apiKey = "INSERT_YOUR_API_KEY_HERE"; // Insert API key here
cout << "Insert city name: ";
getline(cin, city);
string weatherData = getWeatherData(city, apiKey);
if (!weatherData.empty()) {
parseWeatherData(weatherData);
} else {
cout << "Failed to get weather data." << endl;
}
return 0;
}
In the main
function:
- The user is asked to enter a city name.
- Weather data is retrieved using
getWeatherData
. - If the data is successfully retrieved, the weather data is displayed.
Running the Program
To run the application, open a terminal or command prompt in the WeatherApp.cpp
file directory, then compile and run.
g++ WeatherApp.cpp -o WeatherApp -lcurl
./WeatherApp
Troubleshooting Errors
Some common issues:
- Curl error: Make sure
libcurl
is installed. - Incorrect API Key: Check the API key and make sure it is correct.
- Incomplete JSON data: Check your internet connection or the city name entered.
Conclusion
With these steps, you have successfully created a simple weather forecast application using C++. This application can be developed further, for example by adding language selection features, graphical displays, or utilizing UI libraries for a more attractive appearance. Hopefully this article helps!
That’s all the articles from Admin, hopefully useful… Thank you for stopping by…