Published on May 28, 2025
Next.Js E-Commerce Tutorial For Beginners
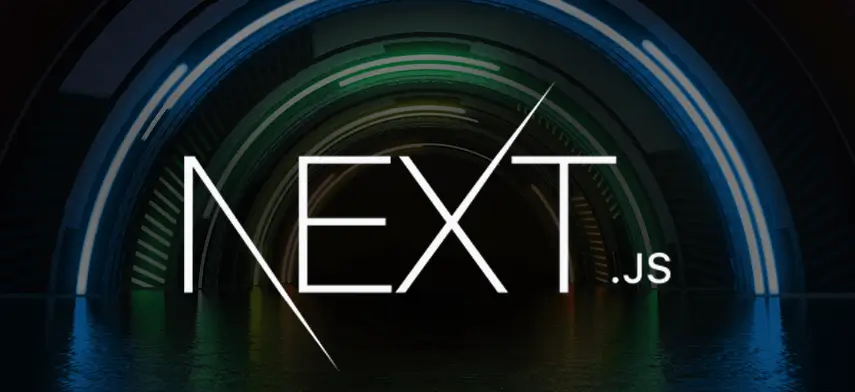
Next.js is a JavaScript framework built on top of React that allows you to create web applications and static sites very efficiently. Created by Vercel, Next.js provides advanced features such as server-side rendering (SSR), static site generation (SSG), API routes, and automatic optimization for speed and SEO. This framework is very popular among front-end developers because of its simplicity and ability to handle various web application development needs automatically.
With Next.js, you can easily create dynamic web applications that are also well-optimized for SEO, considering that Next.js emphasizes server-side rendering and pre-rendering capabilities. This makes it very suitable for building e-commerce sites that require high performance and optimal SEO achievement.
Why Use Next.js for E-Commerce?
When building an e-commerce site, there are several needs and challenges that must be considered. Some of them are:
- Speed and Performance
E-commerce users often need quick access to products and checkout pages. Using Next.js allows for performance optimization by pre-rendering pages, which helps reduce page load times. Additionally, with server-side rendering, Next.js can generate content that is fully rendered on the server, minimizing the time it takes to load data on the client side.
- SEO (Search Engine Optimization)
E-commerce sites need to appear in Google search results to get a lot of organic traffic. Next.js allows for better SEO optimization because it supports server-side rendering by default. With SSR, product content can be rendered on the server and directly available on the HTML page, making it easier for search engines to index it. This provides a major advantage over single-page React applications that only use client-side rendering (CSR), which often makes it difficult for search engines to index content properly.
- Scalability and Flexibility
As your e-commerce business grows, your site needs to be able to handle increased traffic and transactions. Next.js makes it easy to build scalable and flexible applications. With API routes and the ability to perform server-side rendering and static generation simultaneously, you can easily customize and grow your e-commerce site.
- Better User Experience
User experience (UX) is a key factor in e-commerce. Next.js ensures that your application has fast loading times, a responsive interface, and smooth navigation. By supporting features such as lazy loading and image optimization, users will enjoy a smooth and efficient shopping experience.
Benefits of Using Next.js in E-Commerce Development
There are several key benefits that you can enjoy when building an e-commerce site using Next.js:
- Pre-rendering and SEO-friendly
With the ability to perform server-side rendering and static site generation, Next.js makes your site easier to find by search engines. Products and other important content will be rendered faster and can be indexed immediately.
- Optimal User Experience
Site speed is a critical factor in influencing conversions and customer satisfaction. Next.js automatically optimizes application performance with features like lazy loading and resource management.
- API Routes for Backend
Next.js allows you to build APIs directly within your Next.js project using API routes, without the need for a separate server. This makes it easy to manage data, such as products and user transactions, in one place.
- Image and Media Optimization
Ecommerce sites rely on crisp, clear product images. Next.js comes with automatic support for image optimization, reducing file sizes and increasing page speed without sacrificing image quality.
- Efficient State Management
Based on React, Next.js allows for the use of various state management solutions such as Redux or Context API to handle application state, including shopping carts, user status, and other preferences.
- Easy Deployment
Platforms like Vercel allow you to easily deploy your Next.js applications to the cloud, ensuring that your applications are always available and can scale as needed.
With an understanding of the advantages and reasons for choosing Next.js for e-commerce, we will now discuss how to prepare an e-commerce project using Next.js.
Project Preparation
Before we start building an e-commerce application with Next.js, there are several preparatory steps that need to be taken, including installing the necessary software and creating a new Next.js project.
1. System Requirements and Required Tools
To develop an e-commerce site using Next.js, you need to prepare the following tools and software:
Node.js: Next.js runs on top of Node.js, so make sure you have it installed. Use the latest version to get the latest features and improvements.
NPM or Yarn: Package manager to install dependencies. By default, Node.js already includes NPM, but you can also use Yarn as an alternative.
Git: Useful for managing your project version.
Code Editor (VS Code is recommended): Code editor that will be used to write and edit projects.
Modern Browser (Google Chrome or Firefox): To test the appearance and functionality of the application.
Checking Node.js and NPM Installation
Before continuing, make sure Node.js is installed by running the following command in the terminal or command prompt:
node -v
If Node.js is installed, you will see the output in the form of the installed Node.js version, for example:
v18.16.0
To make sure NPM is also installed, run:
npm -v
If successful, the NPM version used will appear, for example:
9.5.1
If Node.js is not installed, you can download and install it from the official website https://nodejs.org .
2. Creating a New Next.js Project
Now that we have all the tools ready, we will create a new Next.js project for e-commerce.
Using Create Next App
The easiest way to start a Next.js project is to use the following command in the terminal:
npx create-next-app@latest next-ecommerce
Or, if you are using Yarn:
yarn create next-app next-ecommerce
This command will create a new Next.js project with a basic configuration. You will be asked to answer a few configuration questions such as:
- Would you like to use TypeScript? → Type
No
if you do not want to use TypeScript (orYes
if you do want to use TypeScript). - Would you like to use ESLint? → Type
Yes
to add linting to your project. - Would you like to use Tailwind CSS? → Type
Yes
if you want to use Tailwind for styling. - Would you like to use “src/” directory? → Type
Yes
to save the code in thesrc
folder. - Would you like to use experimental app directory? → Type
No
(unless you want to try out experimental features).
After the installation process is complete, enter the project directory with the command:
cd next-ecommerce
Then run the application to make sure everything is working properly:
npm run dev
If successful, you should see a message like this:
Local: http://localhost:3000
Open a browser and access localhost:3000
. You should see the default Next.js page.
3. Directory and File Structure in Next.js Project
After the project is successfully created, let’s see the resulting directory structure:
next-ecommerce/
│── node_modules/
│── public/
│── src/
│ │── pages/
│ │ │── _app.js
│ │ │── index.js
│ │── components/
│ │── styles/
│── .gitignore
│── package.json
│── README.md
Explanation of each folder and files:
pages/
→ Contains the main page of the application. Next.js automatically makes every file in this folder a route.public/
→ Folder for storing static assets such as images and icons.components/
→ Place to store reusable components such as headers, footers, and product cards.styles/
→ Folder for CSS and styling filespackage.json
→ Contains project information and dependencies used.
Now that we have a basic Next.js project set up, we will start building the user interface for e-commerce by creating a product page.
User Interface Design
Now that we have a Next.js project set up, the next step is to build the user interface (UI) for our e-commerce application. In this section, we will:
- Create a product page
- Display a list of products
- Add product images and descriptions
- Use Tailwind CSS for styling (optional)
1. Create a Product Page
In the pages/
folder, we will create the main e-commerce page that displays a list of products.
Open the src/pages/index.js
file and change the code to the following:
import Head from "next/head";
export default function Home() {
return (
<div>
<Head>
<title>Next.js E-Commerce</title>
<meta name="description" content="Online store with Next.js" />
</Head>
<h1>Welcome to Online Store</h1>
<p>Discover the best products at the best prices!</p>
</div>
);
}
Code explanation:
<Head>
: Used to add meta tags, which are important for SEO.<h1>
and<p>
: Basic elements for displaying text on the main page.
Now run the server with the command:
npm run dev
Then open a browser and access localhost:3000
, the text “Welcome to the Online Store” should appear.
2. Displaying a List of Products
Next, we will display several products on the main page. For now, we will use dummy product data.
Open the src/pages/index.js
file and change the code to:
import Head from "next/head";
const products = [
{ id: 1, name: "Laptop Gaming", price: 15000000, image: "/laptop.jpg" },
{ id: 2, name: "5G Smartphone", price: 8000000, image: "/smartphone.jpg" },
{ id: 3, name: "Bluetooth Headset", price: 500000, image: "/headset.jpg" },
];
export default function Home() {
returns (
<div>
<Head>
<title>Next.js E-Commerce</title>
<meta name="description" content="Online shop with Next.js" />
</Head>
<h1>Welcome to the Online Store</h1>
<div style={{ display: "flex", gap: "20px", flexWrap: "wrap" }}>
{products.map((product) => (
<div
key={product.id}
style={{
border: "1px solid #ddd",
padding: "10px",
borderRadius: "5px",
width: "200px",
textAlign: "center",
}}
>
<img src={product.image} alt={product.name} width="100%" />
<h2>{product.name}</h2>
<p>Rp {product.price.toLocaleString()}</p>
<button style={{ backgroundColor: "blue", color: "white", padding: "5px 10px" }}>
Add to Cart
</button>
</div>
))}
</div>
</div>
);
}
Code explanation:
- We create an array
products
to store dummy product data. - Use
map()
function to display the product list in a simple card. - The product images are stored in the
public/
folder. Make sure you have the image fileslaptop.jpg
,smartphone.jpg
, andheadset.jpg
inside thepublic/
folder.
Now look back at the browser, the home page will display the product list in card form!
3. Using Tailwind CSS for Styling (Optional)
To make the appearance more attractive, we can use Tailwind CSS. If you selected “Yes” for Tailwind CSS when creating a project, this framework is ready to use. If not, you can install it with the following command:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Then open the tailwind.config.js
file and change it to be like this:
module.exports = {
content: ["./src//.{js,ts,jsx,tsx}"],
theme: {
extend: {},
},
plugins: [],
};
Then, edit the file src/styles/globals.css
and add this line at the beginning:
@tailwindbase;
@tailwind components;
@tailwind utilities;
Now change the code in src/pages/index.js
to use Tailwind CSS:
import Head from "next/head";
const products = [
{ id: 1, name: "Laptop Gaming", price: 15000000, image: "/laptop.jpg" },
{ id: 2, name: "5G Smartphone", price: 8000000, image: "/smartphone.jpg" },
{ id: 3, name: "Bluetooth Headset", price: 500000, image: "/headset.jpg" },
];
export default function Home() {
returns (
<div className="min-h-screen p-6">
<Head>
<title>Next.js E-Commerce</title>
<meta name="description" content="Online shop with Next.js" />
</Head>
<h1 className="text-3xl font-bold text-center">Welcome to Online Store</h1>
<div className="flex flex-wrap justify-center gap-6 mt-6">
{products.map((product) => (
<div
key={product.id}
className="border border-gray-300 p-4 rounded-lg w-64 text-center shadow-lg"
>
<img src={product.image} alt={product.name} className="w-full h-40 object-cover" />
<h2 className="text-xl font-semibold mt-2">{product.name}</h2>
<p className="text-lg text-gray-600">Rp {product.price.toLocaleString()}</p>
<button className="bg-blue-500 text-white px-4 py-2 rounded mt-4 hover:bg-blue-600">
Add to Cart
</button>
</div>
))}
</div>
</div>
);
}
Changes with Tailwind CSS:
- Add margin and padding to make it neater.
- Use flexbox so that the products are arranged properly.
- Add shadow and hover effects to make it more attractive.
Now open the browser, and the product display is more modern and responsive!
Temporary Conclusion
In this section, we have succeeded in:
- Creating a home page with a list of products.
- Displaying products in the form of card with images and prices.
- Using Tailwind CSS to beautify the appearance.
Next, we will add a Shopping Cart feature so that users can add the products they want!
Adding a Shopping Cart Feature
To make e-commerce more interactive, we will add a shopping cart feature. This feature allows users to add products to the cart and see the total price of their purchases.
1. Creating a State to Store Products in the Cart
Because we are using React (Next.js), we can use useState to store a list of products added to the cart.
Open the src/pages/index.js
file and change the code to something like this:
import { useState } from "react";
import Head from "next/head";
const products = [
{ id: 1, name: "Laptop Gaming", price: 15000000, image: "/laptop.jpg" },
{ id: 2, name: "5G Smartphone", price: 8000000, image: "/smartphone.jpg" },
{ id: 3, name: "Bluetooth Headset", price: 500000, image: "/headset.jpg" },
];
export default function Home() {
const [cart, setCart] = useState([]);
const addToCart = (product) => {
setCart([...cart, product]);
};
returns (
<div className="min-h-screen p-6">
<Head>
<title>Next.js E-Commerce</title>
<meta name="description" content="Online shop with Next.js" />
</Head>
<h1 className="text-3xl font-bold text-center">Welcome to Online Store</h1>
<div className="flex flex-wrap justify-center gap-6 mt-6">
{products.map((product) => (
<div key={product.id} className="border border-gray-300 p-4 rounded-lg w-64 text-center shadow-lg">
<img src={product.image} alt={product.name} className="w-full h-40 object-cover" />
<h2 className="text-xl font-semibold mt-2">{product.name}</h2>
<p className="text-lg text-gray-600">Rp {product.price.toLocaleString()}</p>
<button
className="bg-blue-500 text-white px-4 py-2 rounded mt-4 hover:bg-blue-600"
onClick={() => addToCart(product)}
>
Add to Cart
</button>
</div>
))}
</div>
{/ Displays the contents of the basket /}
<div className="mt-8 p-4 border-t border-gray-300">
<h2 className="text-2xl font-bold">Shopping Cart</h2>
{cart.length > 0 ? (
<ul>
{cart.map((item, index) => (
<li key={index} className="flex justify-between p-2 border-b">
<span>{item.name}</span>
<span>Rp {item.price.toLocaleString()}</span>
</li>
))}
</ul>
) : (
<p className="text-gray-500">Shopping cart is still empty.</p>
)}
</div>
</div>
);
}
2. Code Explanation
What’s new in this code?
- Using
useState([])
to store the list of products in the cart. - The
addToCart(product)
function to add products to the cart. - Displaying the contents of the shopping cart below the product list.
Try restarting the server with the command:
npm run dev
Then open a browser, click the Add to Cart button, and see how the product appears in the shopping cart list!
3. Adding Remove Product from Cart Feature
Currently, we can only add products to the cart, but not remove them. Let’s add a remove product from cart feature.
Change the code in the cart section like this:
const removeFromCart = (index) => {
setCart(cart.filter((_, i) => i !== index));
};
Then, in the cart section, add a remove button:
<ul>
{cart.map((item, index) => (
<li key={index} className="flex justify-between p-2 border-b">
<span>{item.name}</span>
<span>Rp {item.price.toLocaleString()}</span>
<button
className="bg-red-500 text-white px-2 py-1 rounded hover:bg-red-600"
onClick={() => removeFromCart(index)}
>
Remove
</button>
</li>
))}
</ul>
Now users can remove products from the cart by clicking the “Remove” button.
4. Displaying Total Price in Cart
To be more informative, we can display total price of all products in the cart.
Add the following code below the cart list:
const totalPrice = cart.reduce((sum, item) => sum + item.price, 0);
Then, display the total price after the list of products in the cart:
{cart.length > 0 && (
<div className="mt-4 font-semibold text-lg">
Total: Rp {totalPrice.toLocaleString()}
</div>
)}
5. Adding Notification when Product Added to Cart
To be more interactive, we can add a simple notification with React state.
Add a message
state for the notification:
const [message, setMessage] = useState("");
Update the addToCart
function to display the notification:
const addToCart = (product) => {
setCart([...cart, product]);
setMessage(`${product.name} successfully added to cart!`);
setTimeout(() => setMessage(""), 2000);
};
Add a notification element below the main page title:
{message && (
<div className="bg-green-200 text-green-800 p-2 rounded-md text-center my-4">
{message}
</div>
)}
Now, every time you add a product to your cart, you will see a temporary notification!
Summary
In this section, we have:
- Added a shopping cart feature with
useState
- Created an “Add to Cart” button for each product
- Displayed a list of products in the shopping cart
- Added a “Remove” button to remove a product from the cart
- Displayed the total price of the products in the cart
- Added a notification when a product is added
Now our e-commerce is starting to feel functional!
Next Steps
For the next step, we can add:
- Checkout page to complete the order
- Saving the cart to local storage so that it remains when the page is refreshed
- Integration with a backend or database (e.g. Firebase or MongoDB)
Adding Checkout & Data Storage Features
Now we will add a checkout feature so that users can complete their orders. We will also save the cart data using Local Storage, so that the products in the cart remain saved even when the page is refreshed.
- Email Spoofing: What It Is, How It Works, and How to Avoid It
- CVE-2024-30085: Privilege Escalation Vulnerability in Windows Cloud Files Mini Filter Driver
- NestJS vs Next.js: A Complete Comparison for Modern App Development
- How to Fix Keyboard Cannot Type or Error
- Understanding Solara Exploit: Definition, Impact, and Prevention Steps
1. Saving Cart Data to Local Storage
Currently, the shopping cart is only stored in React state. If the user refreshes the page, all data will be lost. The solution, we can use Local Storage so that the data remains saved.
Modifying State to be Stored in Local Storage
Open src/pages/index.js
and change the code like this:
import { useState, useEffect } from "react";
import Head from "next/head";
const products = [
{ id: 1, name: "Laptop Gaming", price: 15000000, image: "/laptop.jpg" },
{ id: 2, name: "5G Smartphone", price: 8000000, image: "/smartphone.jpg" },
{ id: 3, name: "Bluetooth Headset", price: 500000, image: "/headset.jpg" },
];
export default function Home() {
const [cart, setCart] = useState([]);
// Load the bucket from Local Storage when first rendering
useEffect(() => {
const savedCart = localStorage.getItem("cart");
if (savedCart) {
setCart(JSON.parse(savedCart));
}
}, []);
// Save the bucket to Local Storage every time it changes
useEffect(() => {
localStorage.setItem("cart", JSON.stringify(cart));
}, [cart]);
const addToCart = (product) => {
setCart([...cart, product]);
};
const removeFromCart = (index) => {
setCart(cart.filter((_, i) => i !== index));
};
const totalPrice = cart.reduce((sum, item) => sum + item.price, 0);
returns (
<div className="min-h-screen p-6">
<Head>
<title>Next.js E-Commerce</title>
<meta name="description" content="Online shop with Next.js" />
</Head>
<h1 className="text-3xl font-bold text-center">Welcome to Online Store</h1>
<div className="flex flex-wrap justify-center gap-6 mt-6">
{products.map((product) => (
<div key={product.id} className="border border-gray-300 p-4 rounded-lg w-64 text-center shadow-lg">
<img src={product.image} alt={product.name} className="w-full h-40 object-cover" />
<h2 className="text-xl font-semibold mt-2">{product.name}</h2>
<p className="text-lg text-gray-600">Rp {product.price.toLocaleString()}</p>
<button
className="bg-blue-500 text-white px-4 py-2 rounded mt-4 hover:bg-blue-600"
onClick={() => addToCart(product)}
>
Add to Cart
</button>
</div>
))}
</div>
{/ Displays the contents of the basket /}
<div className="mt-8 p-4 border-t border-gray-300">
<h2 className="text-2xl font-bold">Shopping Cart</h2>
{cart.length > 0 ? (
<ul>
{cart.map((item, index) => (
<li key={index} className="flex justify-between p-2 border-b">
<span>{item.name}</span>
<span>Rp {item.price.toLocaleString()}</span>
<button
className="bg-red-500 text-white px-2 py-1 rounded hover:bg-red-600"
onClick={() => removeFromCart(index)}
>
Wipe
</button>
</li>
))}
</ul>
) : (
<p className="text-gray-500">The shopping cart is still empty.</p>
)}
{cart.length > 0 && (
<div className="mt-4 font-semibold text-lg">
Total: Rp {totalPrice.toLocaleString()}
</div>
)}
<button
className="bg-green-500 text-white px-4 py-2 rounded mt-4 hover:bg-green-600"
onClick={() => window.location.href = "/checkout"}
>
Checkout
</button>
</div>
</div>
);
}
2. Adding a Checkout Page
Next, we create a checkout page in src/pages/checkout.js
.
Create a new file checkout.js
in the src/pages/
folder, then add the following code:
import { useState, useEffect } from "react";
import Head from "next/head";
export default function Checkout() {
const [cart, setCart] = useState([]);
useEffect(() => {
const savedCart = localStorage.getItem("cart");
if (savedCart) {
setCart(JSON.parse(savedCart));
}
}, []);
const totalPrice = cart.reduce((sum, item) => sum + item.price, 0);
returns (
<div className="min-h-screen p-6">
<Head>
<title>Checkout - Next.js E-Commerce</title>
</Head>
<h1 className="text-3xl font-bold text-center">Checkout</h1>
{cart.length > 0 ? (
<div className="mt-6">
<ul>
{cart.map((item, index) => (
<li key={index} className="flex justify-between p-2 border-b">
<span>{item.name}</span>
<span>Rp {item.price.toLocaleString()}</span>
</li>
))}
</ul>
<div className="mt-4 font-semibold text-lg">Total: Rp {totalPrice.toLocaleString()}</div>
{/ Checkout Form /}
<div className="mt-6">
<h2 className="text-xl font-bold">Payment Details</h2>
<form className="mt-4">
<label className="block">
Full name:
<input type="text" className="border w-full p-2 mt-1 rounded" required />
</label>
<label className="block mt-4">
Shipping address:
<input type="text" className="border w-full p-2 mt-1 rounded" required />
</label>
<label className="block mt-4">
Payment Method:
<select className="border w-full p-2 mt-1 rounded">
<option>Transfer Bank</option>
<option>e-Wallet</option>
<option>Credit Card</option>
</select>
</label>
<button
type="submit"
className="bg-blue-500 text-white px-4 py-2 rounded mt-4 hover:bg-blue-600"
onClick={() => alert("Order successfully created!")}
>
Create Order
</button>
</form>
</div>
</div>
) : (
<p className="text-gray-500 text-center mt-6">Shopping cart is still empty.</p>
)}
</div>
);
}
3. Checkout Test
- Run the server with
npm run dev
- Add products to the cart
- Click “Checkout”
- Fill in the shipping details
- Click “Create Order”, and an alert will appear “Order successfully created!”
Now our online store can complete orders!
Conclusion
- Save the shopping cart to Local Storage
- Add a Checkout page
- Provide a payment form
- Display the total price on the checkout page
Next Steps:
- Integrate payment APIs such as Midtrans or Stripe
- Save order data to a database (Firebase or MongoDB)
Backend Integration & Online Payments in Next.js E-Commerce
Now, our online store can display products, save shopping carts, and complete orders. The next steps are:
- Save order data to the database
- Connect online payments (examples: Midtrans, Stripe, or PayPal)
1. Using Firebase to Store Order Data
To store customer orders safely, we will use Firebase Firestore.
Setup Firebase Firestore
- Open Firebase Console and create a new project.
- Add Firestore Database in the “Build > Firestore Database” menu.
- Create a new collection named
orders
to store customer orders. - Get the Firebase API Configuration, then create a
.env.local
file in the root of the Next.js project and fill it in:
NEXT_PUBLIC_FIREBASE_API_KEY=your_api_key
NEXT_PUBLIC_FIREBASE_AUTH_DOMAIN=your_project.firebaseapp.com
NEXT_PUBLIC_FIREBASE_PROJECT_ID=your_project_id
NEXT_PUBLIC_FIREBASE_STORAGE_BUCKET=your_project.appspot.com
NEXT_PUBLIC_FIREBASE_MESSAGING_SENDER_ID=your_sender_id
NEXT_PUBLIC_FIREBASE_APP_ID=your_app_id
- Install Firebase in the Next.js project
npm install firebase
- Create a
firebase.js
file in thesrc/lib/
folder
import { initializeApp } from "firebase/app";
import { getFirestore } from "firebase/firestore";
const firebaseConfig = {
apiKey: process.env.NEXT_PUBLIC_FIREBASE_API_KEY,
authDomain: process.env.NEXT_PUBLIC_FIREBASE_AUTH_DOMAIN,
projectId: process.env.NEXT_PUBLIC_FIREBASE_PROJECT_ID,
storageBucket: process.env.NEXT_PUBLIC_FIREBASE_STORAGE_BUCKET,
messagingSenderId: process.env.NEXT_PUBLIC_FIREBASE_MESSAGING_SENDER_ID,
appId: process.env.NEXT_PUBLIC_FIREBASE_APP_ID,
};
const app = initializeApp(firebaseConfig);
const db = getFirestore(app);
export { db };
2. Save Order to Firestore
Now we will save the order data to Firestore when the customer checks out.
Open src/pages/checkout.js
and add Firebase Firestore:
import { useState, useEffect } from "react";
import Head from "next/head";
import { db } from "@/lib/firebase";
import { collection, addDoc } from "firebase/firestore";
export default function Checkout() {
const [cart, setCart] = useState([]);
const [name, setName] = useState("");
const [address, setAddress] = useState("");
const [paymentMethod, setPaymentMethod] = useState("Bank Transfer");
const [loading, setLoading] = useState(false);
useEffect(() => {
const savedCart = localStorage.getItem("cart");
if (savedCart) {
setCart(JSON.parse(savedCart));
}
}, []);
const totalPrice = cart.reduce((sum, item) => sum + item.price, 0);
const handleCheckout = async(e) => {
e.preventDefault();
setLoading(true);
try {
const order = {
name,
address,
paymentMethod,
carts,
totalPrice,
status: "Pending",
createdAt: new Date(),
};
// Save to Firestore
await addDoc(collection(db, "orders"), order);
// Clear cart after checkout
localStorage.removeItem("cart");
setCart([]);
alert("Order successfully created!");
} catch (error) {
console.error("Error saving order:", error);
alert("Failed to check out.");
}
setLoading(false);
};
returns (
<div className="min-h-screen p-6">
<Head>
<title>Checkout - Next.js E-Commerce</title>
</Head>
<h1 className="text-3xl font-bold text-center">Checkout</h1>
{cart.length > 0 ? (
<div className="mt-6">
<ul>
{cart.map((item, index) => (
<li key={index} className="flex justify-between p-2 border-b">
<span>{item.name}</span>
<span>Rp {item.price.toLocaleString()}</span>
</li>
))}
</ul>
<div className="mt-4 font-semibold text-lg">Total: Rp {totalPrice.toLocaleString()}</div>
<form className="mt-6" onSubmit={handleCheckout}>
<label className="block">
Full name:
<input
type="text"
className="border w-full p-2 mt-1 rounded"
value={name}
onChange={(e) => setName(e.target.value)}
required
/>
</label>
<label className="block mt-4">
Shipping address:
<input
type="text"
className="border w-full p-2 mt-1 rounded"
value={address}
onChange={(e) => setAddress(e.target.value)}
required
/>
</label>
<label className="block mt-4">
Payment Method:
<select
className="border w-full p-2 mt-1 rounded"
value={paymentMethod}
onChange={(e) => setPaymentMethod(e.target.value)}
>
<option>Bank Transfer</option>
<option>e-Wallet</option>
<option>Credit Card</option>
</select>
</label>
<button
type="submit"
className="bg-blue-500 text-white px-4 py-2 rounded mt-4 hover:bg-blue-600"
disabled={loading}
>
{loading ? "Processing..." : "Create Order"}
</button>
</form>
</div>
) : (
<p className="text-gray-500 text-center mt-6">The shopping cart is still empty.</p>
)}
</div>
);
}
3. Connecting Online Payment API
Next, we can connect Midtrans, Stripe, or PayPal to accept payments.
Example: Using Midtrans
Create an account at Midtrans and get an API Key.
Add the API Key to
.env.local
MIDTRANS_SERVER_KEY=your_midtrans_server_key
MIDTRANS_CLIENT_KEY=your_midtrans_client_key
- Install axios for API communication
npm install axios
- Modify
handleCheckout
to connect to Midtrans
import axios from "axios";
const handleCheckout = async (e) => {
e.preventDefault();
setLoading(true);
try {
const response = await axios.post("/api/payment", {
totalPrice,
});
window.location.href = response.data.redirect_url;
} catch (error) {
console.error("Payment error:", error);
alert("Failed to process payment.");
}
setLoading(false);
};
- Create an API endpoint in
src/pages/api/payment.js
import axios from "axios";
export default async function handler(req, res) {
if (req.method === "POST") {
try {
const response = await axios.post("https://api.sandbox.midtrans.com/v2/charge", {
transaction_details: {
order_id: new Date().getTime(),
gross_amount: req.body.totalPrice,
},
}, {
headers: {
Authorization: `Basic ${Buffer.from(process.env.MIDTRANS_SERVER_KEY).toString("base64")}`,
"Content-Type": "application/json",
},
});
res.status(200).json(response.data);
} catch (error) {
res.status(500).json({ message: "Failed to create transaction" });
}
}
}
Now our online store has a checkout feature with Midtrans!
Deployment & SEO Optimization on Next.js E-Commerce
Now our online store has the following features:
- Display products
- Save shopping cart
- Checkout with Firebase Firestore
- Online payment with Midtrans
Next steps:
- Deploy to Vercel/Netlify
- SEO optimization so that the store appears on Google
- Increase website speed
1. Deploy to Vercel or Netlify
Deploy to Vercel
- Install Vercel CLI (if not)
npm install -g vercel
- Login to Vercel
vercel login
- Deploy the project
vercel
- Set Environment Variable Configuration
- Go to Vercel Dashboard
- Select the project → Settings → Environment Variables
- Add all the
.env.local
that we created earlier
- Done! The online store is now online at namaprojek.vercel.app
Deploy to Netlify
- Install Netlify CLI
npm install -g netlify-cli
- Login to Netlify
netlify login
- Deploy the project
netlify deploy --prod
Add Environment Variables in Netlify Dashboard
Done! Website is ready to be accessed at projectname.netlify.app
2. SEO Optimization for Next.js E-Commerce
To make our website easy to find on Google, do the following optimizations:
1. Add Meta Tags on Each Page
Open src/pages/_app.js
, add Head from Next.js:
import Head from "next/head";
function MyApp({ Component, pageProps }) {
return (
<>
<Head>
<title>Cheap Online Store - Next.js E-Commerce</title>
<meta name="description" content="Buy quality products at the best prices at Cheap Online Store. Fast & safe shipping!" />
<meta name="keywords" content="online store, cheap shopping, next.js e-commerce" />
<meta name="robots" content="index, follow" />
<meta property="og:title" content="Cheap Online Store" />
<meta property="og:description" content="Buy quality products at the best prices at Cheap Online Store." />
<meta property="og:type" content="website" />
<meta property="og:url" content="https://namatoko.com" />
<meta property="og:image" content="https://namatoko.com/logo.png" />
</Head>
<Component {...pageProps} />
</>
);
}
export default MyApp;
2. Use Schema Markup Data Structure
Schema Markup helps Google understand our website.
Open src/pages/index.js
, add JSON-LD Schema
import Head from "next/head";
export default function Home() {
returns (
<div>
<Head>
<script
type="application/ld+json"
dangerouslySetInnerHTML={{
__html: JSON.stringify({
"@context": "https://schema.org",
"@type": "Store",
"name": "Cheap Online Shop",
"url": "https://namatoko.com",
"logo": "https://namatoko.com/logo.png",
"description": "Buy quality products at the best prices.",
"address": {
"@type": "PostalAddress",
"streetAddress": "Jl. Raya No. 123",
"addressLocality": "Jakarta",
"postalCode": "12345",
"addressCountry": "ID"
},
"contactPoint": {
"@type": "ContactPoint",
"telephone": "+62-812-3456-7890",
"contactType": "customer service"
}
})
}}
/>
</Head>
<h1>Welcome to Toko Online Murah</h1>
</div>
);
}
Schema Validation in Google Structured Data Testing Tool
3. Optimize Images with Next.js Image
- Make sure all images use
<Image />
from Next.js
import Image from "next/image";
<Image src="/produk.jpg" alt="Produk" width={500} height={500} />
- Compress images before uploading to the server with TinyPNG .
4. Use Sitemap & Robots.txt
- Install
next-sitemap
to create automatic sitemap
npm install next-sitemap
- Create a
next-sitemap.js
file in the project root
module.exports = {
siteUrl: "https://namatoko.com",
generateRobotsTxt: true,
};
- Add scripts to
package.json
"scripts": {
"postbuild": "next-sitemap"
}
- Run
npm run build && npm run postbuild
- Add to Google Search Console
- Open Google Search Console
- Submit sitemap.xml
3. Increase Website Speed
1. Use Lazy Load for Images
<Image src="/produk.jpg" alt="Produk" width={500} height={500} loading="lazy" />
2. Use CDN for Static Assets
- Use Cloudflare or Vercel CDN for automatic caching.
3. Activate ISR (Incremental Static Regeneration)
- Change
getServerSideProps
togetStaticProps
inindex.js
:
export async function getStaticProps() {
const res = await fetch("https://api.example.com/products");
const products = await res.json();
return {
props: { products },
revalidate: 60, // regenerate page every 60 seconds
};
}
Congrats! Your Next.js online store is online ready and SEO-friendly!
Final Checklist:
- Deploy to Vercel/Netlify
- SEO Optimization
- Increase website speed
Next Steps:
- Add Wishlist feature
- Use Redux for state management
- Integrate Google Analytics & Facebook Pixel
Final Conclusion
Building e-commerce with Next.js provides many benefits, such as high speed, better SEO, and flexibility in backend integration and online payments. In this tutorial, we have covered the detailed steps to create an online store from scratch to ready to use:
- Create a Next.js & Tailwind CSS project for a responsive and modern look.
- Display a list of products with dynamic data using API or database.
- Create a shopping cart feature using React Hooks & Local Storage.
- Save orders to the database using Firebase Firestore.
- Integrate online payments with Midtrans (can also be Stripe or PayPal).
- Deploy the website to Vercel/Netlify so that it can be accessed online.
- Optimize SEO & performance so that the website is easily found on Google.
With proper optimization, a Next.js-based online store can be a fast, secure, and efficient e-commerce solution.
Next Steps:
- Add Wishlist & Product Review features
- Use Redux or Context API for state management
- Integrate Google Analytics & Facebook Pixel for user tracking
Hopefully this tutorial helps! If you have any questions or want to add other features, please discuss.