Published on Dec 18, 2024
Complete Guide to Creating Web Applications with Express.js
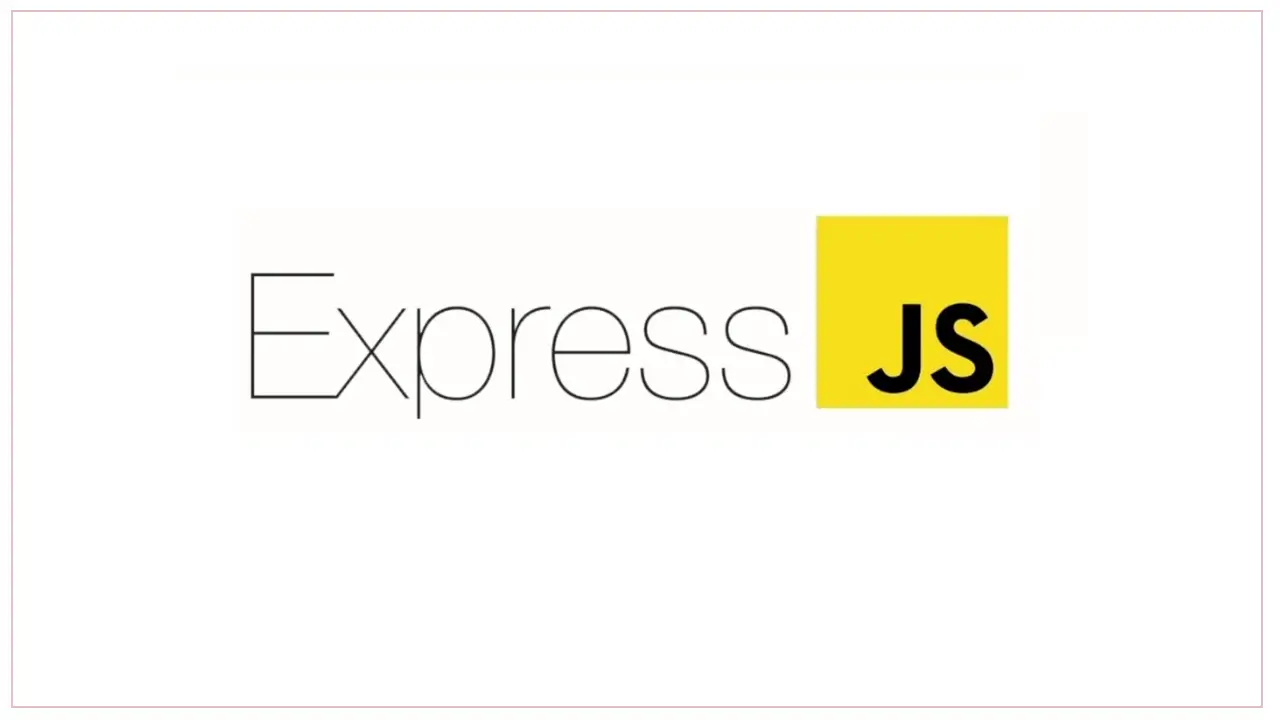
Express.js is one of the most popular Node.js frameworks for building web applications. Using Express, we can easily create HTTP servers, set up routing, and manage various middleware for more dynamic and interactive applications. In this article, we will discuss step by step how to get started with Express.js.
What is Express.js?
Express.js is a minimalist framework for Node.js that makes it easy to develop backend applications. Compared to Node.js alone, Express offers a more organized structure and provides various additional features to organize the application flow. This makes Express a very popular choice for developers to build RESTful APIs and full-stack applications.
Why Use Express.js?
Some reasons why Express is the top choice for many developers:
- Fast and Light: Built on Node.js, Express is very fast and efficient.
- Easy to Learn: Express has comprehensive documentation and a large community.
- Supports Middleware: Middleware makes it easier for us to handle certain tasks such as authentication, logging, and data parsing.
- Compatible with Various Databases: Express can be used with MongoDB, MySQL, PostgreSQL, and other databases.
Preparation
Before you start, make sure that Node.js is installed on your computer. You can check this by typing:
node -v
npm -v
If Node.js and npm are not installed, you can download them from the Official Node.js Website .
Once Node.js is installed, create a new directory for your Express project:
mkdir express-tutorial
cd express-tutorial
Step 1: Installing Express.js
To get started, we’ll create a package.json
file using the following command:
npm init -y
This file will manage your dependencies and project information. Next, install Express.js with the command:
npm install express
Now that Express is successfully installed, we can start writing our first code!
Step 2: Creating a Simple Server
Create a new file called app.js
and add the following code:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
Let’s break down the above code:
const express = require('express');
imports the Express module into the project.const app = express();
creates an instance of the Express app.app.get('/', (req, res) => {...});
handles the GET request on the/
route and sends the response “Hello, Express!”.app.listen(port, () => {...});
creates a server and listens for requests on the specified port (3000 in this example).
To run the server, use the command:
node app.js
Access http://localhost:3000
in your browser, and you should see the message “Hello, Express!”.
Step 3: Adding Routing
Routing is one of the main features of Express. With routing, we can set different responses based on the URL requested by the client. Here is an example of adding multiple routes:
app.get('/about', (req, res) => {
res.send('This is the About page');
});
app.get('/contact', (req, res) => {
res.send('This is the Contact page');
});
Now, we have three routes:
/
for the home page./about
for the “About” page./contact
for the “Contact” page.
Every time you access one of these URLs in a browser, Express will return a response according to the configured routes.
Step 4: Using Middleware
Middleware is a function that executes code between a client request and a server response. Middleware is useful for handling authentication, logging, and other repetitive operations. Here is a simple example of using middleware:
app.use((req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
});
The middleware above logs each incoming request by displaying the HTTP method and URL. next()
is called to proceed to the next middleware or to the appropriate route.
Step 5: Managing Static Files
Express makes it easy to serve static files like images, CSS, and JavaScript. To do this, we can use the built-in middleware express.static()
:
app.use(express.static('public'));
Save your static files in a folder called public
, and now you can access them directly via URL. For example, if you have a file public/style.css
, you can access it at http://localhost:3000/style.css
.
Step 6: Using a Template Engine
To create dynamic pages, we can use a template engine like EJS, Pug, or Handlebars. In this example, we’ll use EJS.
First, install EJS:
npm install ejs
Then, set Express to use EJS as the template engine:
app.set('view engine', 'ejs');
Create a new folder called views
in your project directory, then create an index.ejs
file inside it:
<!DOCTYPE html>
<html>
<head>
<title>Home Page</title>
</head>
<body>
<h1>Welcome to Express!</h1>
</body>
</html>
Now, change your home route to render this template:
app.get('/', (req, res) => {
res.render('index');
});
When you open http://localhost:3000
in your browser, Express will render the index.ejs
template.
Step 7: Create an API Endpoint
Express is very popular for building RESTful APIs. Here’s an example of creating an endpoint to get user data:
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
app.get('/api/users', (req, res) => {
res.json(users);
});
app.get('/api/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
res.json(user);
});
The first endpoint, /api/users
, sends all user data. The second endpoint, /api/users/:id
, sends user data based on the given ID.
Step 8: Handling Errors
To handle errors in Express, we can create a custom middleware to catch and handle errors:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
This middleware will catch all unhandled errors and send an error message to the client.
Conclusion
In this tutorial, we have covered the basics of Express.js, from creating a simple server to using middleware, routing, template engines, and building API endpoints. Express is a powerful and flexible framework that allows us to build backend applications quickly and efficiently.
If you want to deepen your understanding, you can read more documentation or try building a small project with Express. Happy learning and good luck!
That’s all the articles from Admin, hopefully useful… Thank you for stopping by…