Published on May 24, 2025
Complete React JS Tutorial for Beginners
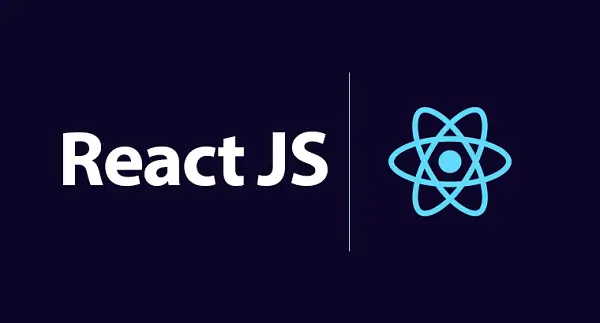
React JS is a JavaScript library used to build interactive and dynamic user interfaces (UI). Developed by Facebook, React has become one of the most popular frontend technologies in the world, used by many large companies such as Instagram, Airbnb, and Netflix.
In this tutorial, we will discuss React from basic to advanced, including how to install it, core concepts, components, state, props, to state management with Redux.
What is React JS?
React is a JavaScript library for building component-based user interfaces. With React, developers can create flexible, easy-to-manage, and efficiently updateable UIs using Virtual DOM.
Advantages of React JS
Virtual DOM: React uses Virtual DOM to improve application performance by only updating the changed parts of the UI.
Reusable Components: React allows the creation of reusable components.
Unidirectional Data Flow: Data in React flows in one direction, making it easy to debug and maintain code.
Large Ecosystem: With a large community, React has many libraries and tools.
React Setup & Installation
Before you start learning React, make sure you have Node.js and npm installed on your computer.
Install Node.js and npm
You can download and install Node.js from its official website:
Download Node.jsAfter installing Node.js, check the version with the following command:
node -v
npm -v
Create a React Project with Create React App
To start a React project, use the following command:
npx create-react-app my-app
cd my-app
npm start
After the command is executed, React will create a new project and start the development server.
React Folder Structure
Once the project is created, the folder structure will look like this:
my-app/
|-- node_modules/
|-- public/
|-- src/
|-- App.js
|-- index.js
|-- package.json
src/
: Where the main React files are, including the main component (App.js
).public/
: Contains static files such asindex.html
.package.json
: Contains project information and dependencies used.
- Brainfuck: A Unique and Challenging Minimalist Programming Language
- Types of Security Encryption: Definition, How It Works, and Examples
- CVE-2024-30085: Privilege Escalation Vulnerability in Windows Cloud Files Mini Filter Driver
- Using BreezyFlow Tools: Micro SaaS Solutions for SMEs
- What is Pathways Language Model (PaLM)?
React Basics
JSX (JavaScript XML)
JSX is a JavaScript extension that allows us to write HTML inside JavaScript:
const element = <h1>Hello, React!</h1>;
Components in React
React uses the concept of components, which are small, reusable pieces of UI. There are two main types of components:
Functional Components
These components are created with JavaScript functions:
function Greeting() {
return <h1>Hello, React!</h1>;
}
Class-Based Components
These components are created with JavaScript classes:
class Greeting extends React.Component {
render() {
return <h1>Hello, React!</h1>;
}
}
State and Props
State in React
State is an object that stores data in a component:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Add</button>
</div>
);
}
Props in React
Props (Properties) are used to pass data between components:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
Using this component:
<Greeting name="John" />
Event Handling in React
React supports event handling like JavaScript:
function Button() {
function handleClick() {
alert('Button clicked!');
}
return <button onClick={handleClick}>Click Me</button>;
}
State Management with Redux
Redux is a library for managing global state in React applications.
Installing Redux
npm install redux react-redux
Creating a Redux Store
import { createStore } from 'redux';
const initialState = { count: 0 };
function counterReducer(state = initialState, action) {
switch (action. type) {
case 'INCREMENT':
return { count: state.count + 1 };
default:
return state;
}
}
const store = createStore(counterReducer);
export defaultstore;
Using Redux in Components
import { useSelector, useDispatch } from 'react-redux';
function Counter() {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
returns (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>Add</button>
</div>
);
}
Conclusion
In this tutorial, we have covered the basics of React, from installation to using Redux. React is a very flexible technology and is suitable for building modern web applications. By understanding key concepts such as JSX, state, props, and event handling, you can start building React applications with more confidence.
For further learning, you can explore advanced Hooks, React Router, and backend integration.