Published on Dec 18, 2024
Last updated on Apr 11, 2025
Recognizing and Preventing Exploits in Laravel
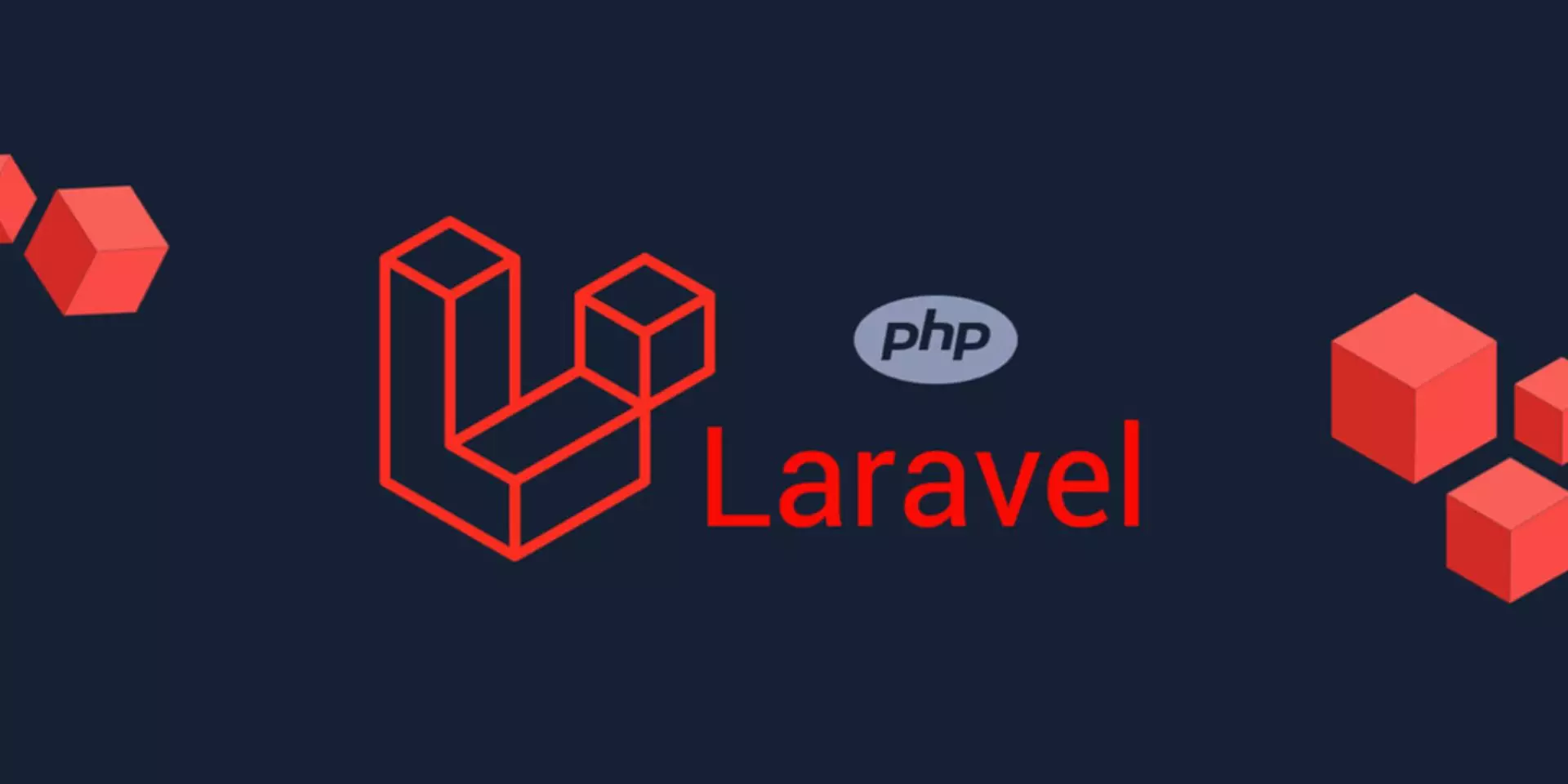
Laravel is one of the most popular PHP frameworks in the world, especially among web developers because of its ease of use, good performance, and the security it provides. Laravel is constantly updated to add the latest security features, but no system is completely free from security holes. In this article, we will discuss the various types of exploits that can occur in Laravel, how to recognize them, and steps to prevent them.
1. Types of Exploits in Laravel
Exploits are the act of taking advantage of vulnerabilities in an application or system to gain unauthorized access or damage the system. In Laravel, some common types of exploits include:
SQL Injection: SQL Injection is an exploitation technique where an attacker attempts to insert SQL code through user input, thereby accessing or modifying unauthorized data.
Cross-Site Scripting (XSS): XSS is a form of attack where an attacker inserts malicious scripts into a website that will then be executed in the user’s browser.
Cross-Site Request Forgery (CSRF): CSRF is an attack where an attacker tricks a user into performing a specific action on a website without the user’s knowledge.
Remote Code Execution (RCE): RCE allows an attacker to execute code on the victim’s server, which can cause serious damage.
2. How SQL Injection Works and How to Prevent It in Laravel
SQL Injection occurs when user input is inserted into an SQL query without going through the sanitization process. For example, if your application accepts user input and uses it in a SQL query without proper validation, an attacker can insert their own SQL commands.
Prevention:
- Using Query Builder or Eloquent ORM: Laravel by default uses Query Builder and Eloquent ORM which automatically sanitize user input. By using this feature, the risk of SQL Injection can be minimized.
// Example of using Eloquent ORM
$user = User::where('email', $email)->first();
Input Validation: Make sure all user input is validated before it is used in a SQL query or other operation.
Use Bind Parameters: If you are using raw queries, make sure to use bind parameters so that user input is not directly inserted into the SQL query.
DB::select('SELECT FROM users WHERE email = ?', [$email]);
3. Dealing with Cross-Site Scripting (XSS) in Laravel
XSS occurs when an attacker injects malicious script into a website that is then executed in the user’s browser. Laravel provides several ways to protect your application from these attacks.
Prevention:
- Escape Output: Laravel uses Blade as its templating engine, which by default escapes potentially malicious content using the
{{ }}
method. Be sure to always use this syntax to prevent user-generated content from being executed directly as HTML.
<div>{{ $user->name }}</div>
- Use
{!! !!}
with Caution: The{!! !!}
syntax allows unescaped output. Only use this if you are absolutely sure that the content is safe. - Data Sanitization: If your application allows HTML input from the user, consider using a library like
HTMLPurifier
to sanitize the data from malicious elements.
4. Prevent Cross-Site Request Forgery (CSRF)
CSRF is an attack where an attacker tricks a user into performing an unwanted action on a website. Laravel provides built-in CSRF protection that can prevent this attack.
Prevention:
- Use CSRF Middleware: Laravel includes CSRF middleware by default, which validates every POST, PUT, DELETE, or PATCH request to ensure that it is legitimate. Make sure not to disable this middleware.
Route::post('/post', function () {
// Logic to handle post requests
})->middleware('csrf');
- Use CSRF Token in Forms: When creating forms, use the CSRF token generated by Laravel to ensure that the form can only be submitted by legitimate users.
<form method="POST" action="/post">
@csrf
<input type="text" name="title">
<button type="submit">Submit</button>
</form>
5. Overcoming Remote Code Execution (RCE)
RCE is a type of attack where an attacker can execute code on the server. This usually happens due to a vulnerability in the system or the use of malicious functions that can execute system commands.
Prevention:
Avoid Using
eval()
Function: Never use theeval()
function or similar functions that allow code execution at runtime.Restrict File Access and Permissions: Make sure that your application does not grant excessive permissions to files and directories. Use strict file permissions to limit attacker access.
Use Strong Input Validation: If your application accepts input from the user, make sure that the input is properly validated and there is no room for malicious code to be injected.
6. Using Laravel Security Features to Minimize Risk
Laravel has provided various built-in security features that can be used to prevent exploitation. Here are some features that can be utilized:
- Encryption: Laravel supports data encryption to protect sensitive data stored on the server.
$encrypted = encrypt('secret data');
$decrypted = decrypt($encrypted);
- Laravel Auth: Laravel provides a built-in authentication system that allows easy management of logins and permissions. This helps protect applications from unauthorized access.
- Rate Limiting: The rate limiting feature helps prevent brute force attacks by limiting the number of requests from a single IP source.
Route::middleware(['auth', 'throttle:10,1'])->group(function () {
// routes that require rate limiting
});
7. Conduct Periodic Security Audits
It is important to regularly perform security audits on your application. Some steps that can be taken include:
- Use Dependency Scanner: Make sure all the packages you use are updated to the latest version. Laravel has a
composer audit
feature that can help detect vulnerabilities in packages. - Penetration Testing: Perform penetration testing on your application to find potential security holes that may have been missed.
- Logging and Monitoring: Make sure you enable logging in Laravel to track suspicious activity and evaluate the logs periodically.
Conclusion
Laravel is a framework that offers various built-in security features, but it still requires attention from developers to keep applications secure. By recognizing various types of exploits such as SQL Injection, XSS, CSRF, and RCE, and understanding how to prevent them, we can build more secure and reliable applications. The combination of good coding practices, Laravel security features, and regular security audits are the keys to protecting applications from potential dangerous exploits.
That’s all the articles from Admin, hopefully useful… Thank you for stopping by…