Published on May 24, 2025
Understanding Python in Programming
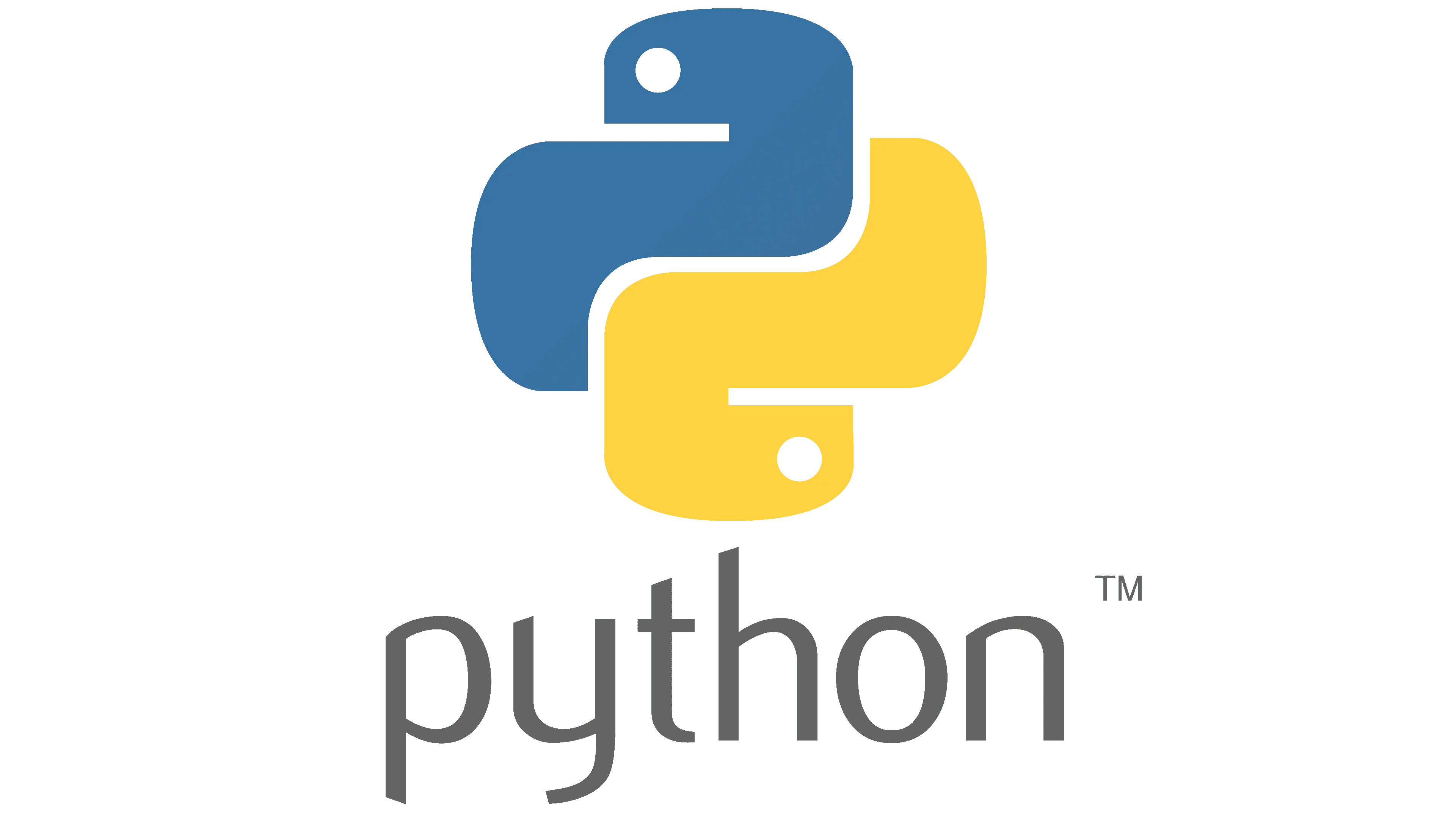
Python is a very popular and versatile programming language, known for its clean syntax and ease of use. Developed by Guido van Rossum and first released in 1991, Python has become one of the leading programming languages in the world of information technology. The language offers a variety of features that make it attractive to developers ranging from beginners to experienced professionals.
Python Advantages
- Clean and Readable Syntax: One of Python’s main attractions is its intuitive syntax. Python code is designed to be easy to read and understand, even for those who are just starting to learn programming. This allows programmers to write cleaner, more efficient code that is free from errors.
- Flexibility and Versatility: Python is used in a variety of applications, from web development, data analysis, artificial intelligence (AI), to scripting and automation. With extensive libraries and frameworks such as Django, Flask, Pandas, and TensorFlow, Python can be used to complete a variety of projects.
- Active Community: Python has a large and active developer community. This means there are plenty of resources, such as tutorials, forums, and libraries, that can help developers solve problems and improve their skills.
- Platform-Independent: Python can run on multiple platforms, including Windows, macOS, and Linux. This makes it easy for developers to work in different environments without having to change the code significantly.
Uses of Python in Different Fields
- Web Development: With frameworks like Django and Flask, Python allows developers to create powerful and efficient web applications. These frameworks offer a variety of built-in features that speed up the development process.
- Data Science and Data Analytics: Python is the language of choice for data analysis and data science. Libraries like Pandas and NumPy make it easy to manipulate and analyze data, while Matplotlib and Seaborn are used for data visualization.
- Artificial Intelligence and Machine Learning: Python is very popular in the fields of artificial intelligence (AI) and machine learning. Libraries like TensorFlow, Keras, and PyTorch provide the necessary tools to build and train AI models.
- Automation and Scripting: Python is often used to write scripts that automate routine tasks. This can include tasks such as file processing, system management, and fetching data from the web.
How to Learn Python From Scratch?
Learning Python from scratch can be a very rewarding and rewarding experience, considering that the language is designed with easy-to-understand syntax and a wide range of applications. Here is a step-by-step guide that you can follow to start your Python learning journey:
1. Understand the Basics of Programming
Before you start learning Python, it’s a good idea to understand some basic programming concepts, such as:
- Variables and Data Types: How to store and manage data in a program.
- Basic Operations: Arithmetic, comparison, and logical operations.
- Control Structures: Using conditional statements (
if-else
) and loops (for
,while
).
2. Installing Python
To start coding with Python, you need to install the Python interpreter on your computer. Follow these steps:
- Download Python: Visit the official Python website at python.org and download the latest version.
- Install Python: Follow the installation instructions according to your operating system. Make sure to check “Add Python to PATH” during installation.
3. Choose an Editor or IDE
An editor or IDE (Integrated Development Environment) is a tool used to write and run Python code. Some popular choices include:
- IDLE: The default editor that comes with Python.
- Visual Studio Code: A powerful source code editor with many extensions for Python.
- PyCharm: A Python-specific IDE that offers a variety of advanced features.
4. Learn Python Syntax
Start by understanding the basic syntax of Python, including:
- Variables and Data Types: Integers, floats, strings, and lists.
- Operators: Arithmetic, logical, and comparison operators.
- Functions: How to define and call functions.
5. Take Online Courses and Tutorials
Here are some helpful resources for learning Python:
- Online Courses: Platforms like Coursera, edX, and Udemy offer Python courses for beginners.
- Interactive Tutorials: Sites like Codecademy and Learn Python offer interactive tutorials that help you learn by doing.
6. Practice Your Code
Practice is key to learning programming. Try small projects to apply what you’ve learned, such as:
- Simple Calculator Program: Calculate basic operations.
- Notes App: Store and organize notes.
- Simple Games: Like Guess the Number or tic-tac-toe.
7. Use Documentation and Community Resources
Python has very comprehensive documentation. If you face any issues or need further explanation, you can refer to:
- Official Python Documentation: docs.python.org
- Forums and Communities: Like Stack Overflow and Reddit.
8. Learn Libraries and Frameworks
Once you are comfortable with the basics of Python, exploring more complex libraries and frameworks can be the next step:
- Pandas and NumPy: For data analysis.
- Django and Flask: For web development.
- TensorFlow and Keras: For artificial intelligence and machine learning.
9. Keep Learning and Experimenting
Programming is an ever-evolving skill. Always look for new challenges and more complex projects to improve your skills. Join a developer community and stay updated with the latest developments in Python and related technologies.
10. Join an Open Source Project
Once you are confident enough with your skills, trying to contribute to an open source project can provide valuable experience and an opportunity to collaborate with other developers.
What are Some Simple Python Projects?
Here are some simple project examples that you can try to practice your Python skills. These projects are suitable for beginners and will help you understand the basic concepts in Python.
1. Simple Calculator
Create a calculator application that can perform basic arithmetic operations such as addition, subtraction, multiplication, and division.
Features:
- Select the desired operation.
- Enter two numbers.
- Display the result of the operation.
Example Code:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a b
def divide(a, b):
return a / b
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
options = input("Enter choices (1/2/3/4): ")
number1 = float(input("Enter first number: "))
number2 = float(input("Enter second number: "))
if choice == '1':
print(f"Result: {add(number1, number2)}")
elif choice == '2':
print(f"Result: {subtract(number1, number2)}")
elif choice == '3':
print(f"Result: {times(number1, number2)}")
elif options == '4':
print(f"Result: {divide(number1, number2)}")
else:
print("Invalid choice")
2. Word Counter Program
Create an application that counts the number of words in a text entered by the user.
Features:
- Enter text.
- Count and display the number of words.
Example Code:
def countwords(text): words = text.split()
return len(words) text = input("Enter text: ")
count_words = count_words(text)
print(f"Number of words: {number_of_words}")
3. Guess the Number Game
Create a simple game where the user has to guess a number randomly selected by the computer.
Features:
- The computer randomly selects a number within a given time period or range.
- The user guesses a number.
- Provide feedback whether the guess is too high or too low.
Sample Code:
import random
secret_number = random.randint(1, 100)
guess = None
while guess != secret_number:
guess = int(input("Guess a number between 1 and 100: "))
if guess < secret_number:
print("Guess too low.")
elif guess > secret_number:
print("Guess too high.")
else:
print("Congratulations! You guessed the number correctly.")
4. Simple Note-Taking Program
Create a simple note-taking application that allows the user to add, view, and delete notes.
Features:
- Add notes.
- View all notes.
- Delete notes by number.
Example Code:
notes = []
def display_notes():
if not notes:
print("No notes.")
else:
for i, item in enumerate(notes, 1):
print(f"{i}. {item}")
def add_notes(text):
notes.append(text)
def delete_notes(index):
if 0 < index <= len(notes):
notes.pop(index - 1)
else:
print("Invalid note number.")
while True:
print("\nMenu:")
print("1. Add note")
print("2. Show note")
print("3. Delete note")
print("4. Exit")
pilihan = input("Select menu (1/2/3/4): ")
if pilihan == '1':
teks = input("Enter note: ")
add_note(text)
elif choice == '2':
show_note()
elif choice == '3':
show_note()
index = int(input("Enter the note number to delete: "))
delete_note(index)
elif choice == '4':
break
else:
print("Invalid choice.")
5. Temperature Converter
Create an application that can convert temperature from Celsius to Fahrenheit and vice versa.
Features:
- Select the conversion type (Celsius to Fahrenheit or Fahrenheit to Celsius).
- Enter the temperature.
- Display the conversion result.
Example Code:
def celsius_to_fahrenheit(celsius):
return (celsius 9/5) + 32
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) 5/9
print("Select temperature conversion:")
print("1. Celsius to Fahrenheit")
print("2. Fahrenheit to Celsius")
options = input("Enter choice (1/2): ")
if options == '1':
temperature_celsius = float(input("Enter temperature in Celsius: "))
temperature_fahrenheit = celsius_to_fahrenheit(temperature_celsius)
print(f"Temperature in Fahrenheit: {temperature_fahrenheit}")
elif options == '2':
temperature_fahrenheit = float(input("Enter the temperature in Fahrenheit: "))
temperature_celsius = fahrenheit_to_celsius(temperature_fahrenheit)
print(f"Temperature in Celsius: {temperature_celsius}")
else:
print("Invalid choice")
6. Shopping List Program
Create an application that allows users to add, view, and delete items from a shopping list.
Features:
- Add items to the list.
- View all items in the list.
- Delete items from the list.
Example Code:
shopping_list = []
def display_list():
if not shopping_list:
print("Shopping list is empty.")
else:
print("Shopping list:")
for item in shopping_list:
print(f"- {item}")
def add_item(item):
shopping_list.append(item)
def delete_item(item):
if item in shopping_list:
shopping_list.remove(item)
else:
print("Item not found in list.")
while True:
print("\nMenu:")
print("1. Add item")
print("2. Show shopping list")
print("3. Remove item")
print("4. Exit")
pilihan = input("Select menu (1/2/3/4): ")
if pilihan == '1':
item = input("Enter an item to add: ")
add_item(item)
elif choice == '2':
show_list()
elif choice == '3':
item = input("Enter an item to remove: ")
remove_item(item)
elif choice == '4':
break
else:
print("Invalid choice.")
These projects are designed to help you understand the basic concepts of Python while providing a rewarding challenge. Good luck!
That’s all the articles from Admin, hopefully useful… Thank you for stopping by…