The Complete Guide to Firebase Database
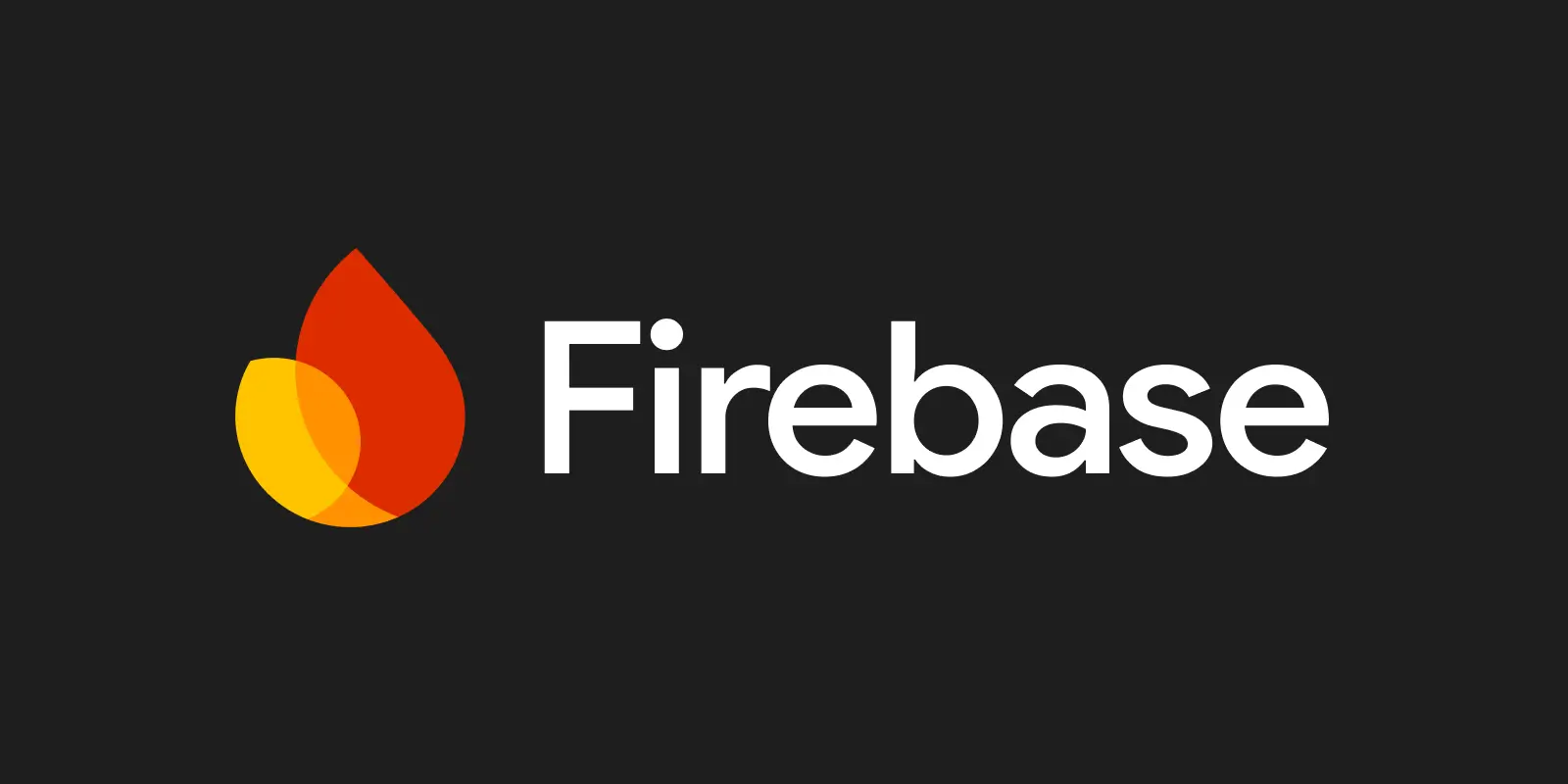
Firebase is an application development platform provided by Google, which provides various backend services to support modern application development. One of the core services of Firebase is Firebase Realtime Database and Firestore. This article will discuss in detail about what is Firebase database, the difference between Realtime Database and Firestore, and how to use it.
What is Firebase Database?
Firebase Database is a database service that allows application developers to store and synchronize data in real-time. Firebase provides two main types of databases:
- Firebase Realtime Database
Firebase Realtime Database is a cloud-based NoSQL database that allows data to be stored in JSON format and synchronizes that data in real-time to all connected clients. This database is suitable for applications that require live data synchronization, such as chat applications or location tracking.
- Cloud Firestore
Cloud Firestore is an extension of Realtime Database. Firestore offers more features, including more complex queries, better scalability, and easier integration with other Google Cloud services.
Firebase Database is designed to make it easy for developers to manage app data with little to no need to build backend servers.
Key Firebase Database Features
Firebase Database has several key features that make it an attractive choice for app developers:
- Real-Time Sync
Firebase Realtime Database allows data to be synced across user devices instantly. This is especially useful for apps like chat, multiplayer games, or collaboration apps.
- Scalability
Firebase supports apps from small to large. With Firestore, developers can handle millions of users while maintaining app performance.
- Security
Firebase comes with JSON-based security rules that allow developers to easily set user access rights. These rules ensure that data can only be accessed by users who have permissions.
- Offline Mode
Firebase Database supports offline mode, which allows apps to continue working even if users lose their internet connection. Data will be resynced when the internet connection is restored.
- Easy Integration
Firebase can be easily integrated with various platforms such as Android, iOS, and web-based applications. Firebase also provides SDKs for various programming languages.
- Fast Data Processing
Firebase allows instant data retrieval and storage, which enhances the user experience in the application.
Firebase Realtime Database vs Cloud Firestore
Although both are database services provided by Firebase, Firebase Realtime Database and Cloud Firestore have fundamental differences. Here is a comparison between the two:
Features | Firebase Realtime Database | Cloud Firestore |
---|---|---|
Data Structures | JSON | Documents and Collections |
Queries | Limited | Complex queries with filters |
Real-Time Sync | Yes | Yes |
Scalability | Suitable for small to medium applications | Designed for large-scale applications |
Offline Mode | Supported | Supported |
Cost | Cheaper for small data | More expensive but more flexible |
Which is Right for You?
If you’re building an app that requires real-time data synchronization with simple data structures, Firebase Realtime Database is a great choice. However, if your app requires high scalability, complex queries, and better integration with other cloud services, Cloud Firestore is a better choice.
- Sinkclose Vulnerability: Definition, Causes, Impacts, and How to Overcome It
- How to Find Laptop Battery Cycle Count on Kali Linux?
- Computer Network System for Data Sharing
- Understanding Databases and Their Various Importance
- What Is HeyGen AI: Understanding Technology and Its Benefits in the Digital Age
How Firebase Realtime Database Works
Firebase Realtime Database uses an event-driven approach to synchronizing data between the client and server. Here’s how it works:
- Storing Data
Data is stored in JSON format in the Firebase Realtime Database.
- Listening for Data Changes
Clients can subscribe to a specific path in the database to listen for data changes in real time. When data is updated, Firebase pushes the new data to all connected clients.
- Synchronization
Firebase ensures that all clients have the latest data, even if they are using different devices or platforms.
- Offline Mode
When a client loses internet connection, Firebase temporarily stores data on the device and will resync it once the connection is restored.
Using Firebase Database
1. Getting Started
To get started with Firebase Database, the first step is to set up a Firebase project. Here are the steps:
-
Open the Firebase Console .
-
Create a new project.
-
Add an app to your project (Android, iOS, or Web).
-
Follow the Firebase SDK installation guide for your chosen platform.
2. Storing Data
Firebase Database allows you to store data easily. Here is a code sample for storing data in Firebase Realtime Database using JavaScript:
import { getDatabase, ref, set } from "firebase/database";
const db = getDatabase();
set(ref(db, 'users/' + userId), {
username: "JohnDoe",
email: "john@example.com",
profile_picture: "https://example.com/john.jpg"
});
3. Reading Data
To read data from Firebase, you can use the onValue
method:
import { getDatabase, ref, onValue } from "firebase/database";
const db = getDatabase();
const userRef = ref(db, 'users/' + userId);
onValue(userRef, (snapshot) => {
const data = snapshot.val();
console.log(data);
});
4. Setting Security Rules
Firebase Realtime Database uses JSON-based security rules to manage user access. Here’s an example of a simple security rule:
{
"rules": {
"users": {
"$uid": {
".read": "$uid === auth.uid",
".write": "$uid === auth.uid"
}
}
}
}
The above rule ensures that each user can only read and write their own data.
Firebase Database Use Case Studies
Firebase Database is used in a variety of applications, including:
- Chat Apps
Firebase Realtime Database is an ideal solution for chat apps because of its ability to sync messages in real-time.
- Location Tracking
Apps like ride-hailing or shipment tracking use Firebase to update location in real time.
- Collaboration Apps
Firebase allows multiple users to work together in real-time, like a collaborative document or digital whiteboard.
- Multiplayer Games
Firebase Realtime Database enables real-time data synchronization between players in a game.
Conclusion
Firebase Database is a powerful tool for building modern applications with real-time data synchronization and high scalability requirements. With two primary options, Firebase Realtime Database and Cloud Firestore, developers have the flexibility to choose the solution that best suits their application needs. With features like real-time sync, offline mode, and customizable security, Firebase Database is a viable option for today’s app development.
That’s all the articles from Admin, hopefully useful… Thank you for stopping by…