SQL Injection: Definition, Dangers, and How to Prevent It
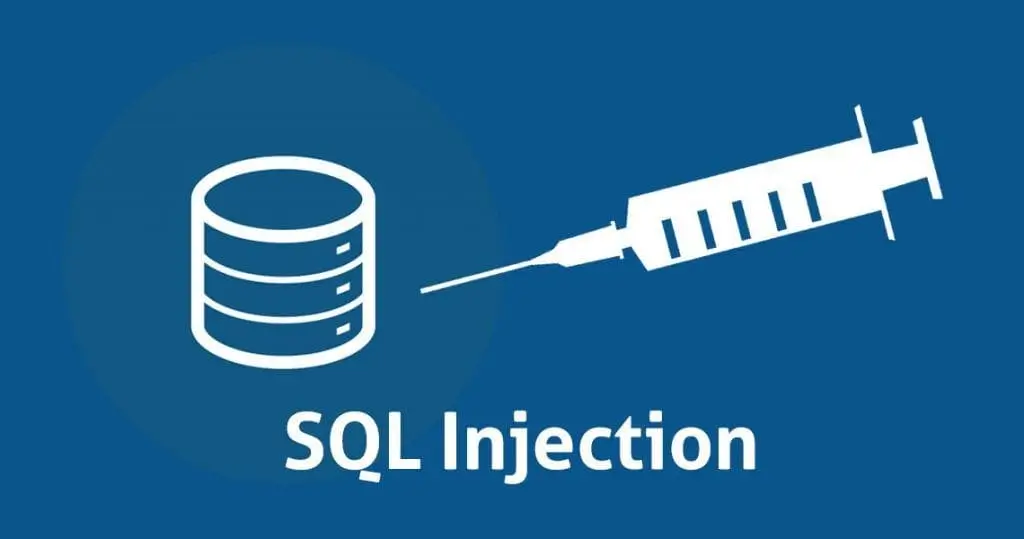
SQL Injection is an attack technique that exploits vulnerabilities in SQL (Structured Query Language) based applications. With this attack, an attacker can insert or ‘inject’ malicious SQL commands into queries created by the application. As a result, the attacker can gain unauthorized access to the database, change, or even delete data.
How SQL Injection Works
SQL Injection attacks usually occur when a web application accepts input from the user and immediately processes it in SQL commands without adequate validation or sanitization. For example, if an application has a login form that checks the user’s credentials through a query such as:
SELECT * FROM users WHERE username = 'input_user' AND password = 'input_pass';
If user input is not validated, an attacker can inject malicious code, such as:
username: ' OR '1'='1
password: ' OR '1'='1
This would change the query to:
SELECT * FROM users WHERE username = '' OR '1'='1' AND password = '' OR '1'='1';
The above query is always true and allows an attacker to log into the application without valid credentials.
SQL Injection Dangers
-
Data Theft: Attackers can access sensitive information such as user personal data, credit card numbers, etc.
-
Data Alteration: Attackers can change or delete critical data, causing significant losses to the business.
-
System Compromise: With access to the database, attackers can gain further access to servers and other systems.
-
Financial Loss: SQL Injection attacks can cause significant financial losses through data theft and recovery costs.
-
Reputational Damage: Security incidents can damage a company’s reputation and reduce customer trust.
SQL Injection Prevention Methods
- Use Parameterized Queries: Instead of constructing queries directly from user input, use parameterized queries or prepared statements which automatically handle input as data, not commands.
Example of using prepared statements in PHP:
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ? AND password = ?");
$stmt->bind_param("ss", $input_user, $input_pass);
$stmt->execute();
- Validate and Sanitize Input: Validate and sanitize all input from the user to ensure that only expected data is received.
Example of input validation in PHP:
$input_user = filter_input(INPUT_POST, 'username', FILTER_SANITIZE_STRING);
$input_pass = filter_input(INPUT_POST, 'password', FILTER_SANITIZE_STRING);
- Proper Use of Access Rights: Limit user access rights to the database. Do not use a database account with high access rights for a web application.
Example of minimal access rights configuration in MySQL:
GRANT SELECT, INSERT, UPDATE, DELETE ON database_name.* TO 'user'@'host' IDENTIFIED BY 'password';
-
Update and Patch Regularly: Always update your systems and applications with the latest patches to close known vulnerabilities.
-
**WAF (Web Application Firewall): Use WAF to automatically detect and prevent SQL Injection attacks.
Conclusion
SQL Injection is a serious threat to web application security. By understanding how it works and its impact, and implementing the right preventive measures, you can protect your applications from these attacks. Security is a long-term investment that is essential to maintaining data integrity and user trust.
That’s all the articles from Admin, hopefully useful… Thank you for stopping by…